vxworks printf到指定shell
时间: 2023-08-16 22:06:40 浏览: 153
在 VxWorks 操作系统中,可以使用 `tyWrite()` 函数将输出信息发送到指定的串口设备,实现将 `printf()` 的输出信息输出到指定的 shell。以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <ioLib.h>
#include <tyLib.h>
int main()
{
int fd = open("/tyCo/1", O_WRONLY, 0);
if (fd == ERROR) {
perror("open");
return ERROR;
}
// 将 printf 输出到串口设备
int old_stdout = ioGlobalStdGet(1);
ioGlobalStdSet(1, fd);
printf("Hello, serial port!\n");
ioGlobalStdSet(1, old_stdout);
close(fd);
return OK;
}
```
在该示例中,通过 `open()` 函数打开 `/tyCo/1` 这个串口设备文件,然后将 `printf()` 的输出重定向到该设备文件。需要注意的是,在 VxWorks 中,标准输出的文件描述符为 1,因此需要使用 `ioGlobalStdSet()` 函数将标准输出重定向到指定的文件描述符,完成输出后,需要将标准输出恢复为原来的设置,否则可能会影响后续的输出。
需要注意的是,在使用 `tyWrite()` 函数发送数据时,需要对数据进行缓存,否则可能会出现数据不完整或丢失的情况。以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <ioLib.h>
#include <tyLib.h>
#include <string.h>
#define MAX_BUF_SIZE 1024
int main()
{
int fd = open("/tyCo/1", O_WRONLY, 0);
if (fd == ERROR) {
perror("open");
return ERROR;
}
char buf[MAX_BUF_SIZE];
memset(buf, 0, sizeof(buf));
sprintf(buf, "Hello, serial port!\n");
int len = strlen(buf);
int bytes_written = 0;
while (bytes_written < len) {
int ret = tyWrite(fd, buf + bytes_written, len - bytes_written);
if (ret == ERROR) {
perror("tyWrite");
break;
}
bytes_written += ret;
}
close(fd);
return OK;
}
```
在该示例中,先将需要发送的数据存入到一个缓冲区中,然后使用循环的方式将缓冲区中的数据发送到指定的串口设备中,直到所有数据都被发送完毕。需要注意的是,在使用 `tyWrite()` 函数发送数据时,需要判断返回值,如果返回值为 `ERROR`,则表示发送失败,需要及时处理。
阅读全文
相关推荐



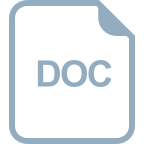

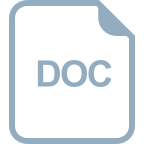

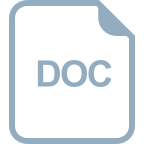










