输入两组点云数据,分别求其凸包和交集的面积的C++代码有吗?
时间: 2024-02-13 19:00:22 浏览: 23
是的,以下是使用C++实现求两组点云数据的凸包和交集面积的代码:
```cpp
#include <iostream>
#include <algorithm>
#include <vector>
#include <cmath>
using namespace std;
struct Point {
double x, y;
Point() {}
Point(double x, double y) : x(x), y(y) {}
bool operator<(const Point& p) const {
return x < p.x || (x == p.x && y < p.y);
}
double distance(const Point& p) const {
double dx = x - p.x;
double dy = y - p.y;
return sqrt(dx * dx + dy * dy);
}
double cross(const Point& p) const {
return x * p.y - y * p.x;
}
Point operator-(const Point& p) const {
return Point(x - p.x, y - p.y);
}
};
double cross(const Point& O, const Point& A, const Point& B) {
return (A.x - O.x) * (B.y - O.y) - (A.y - O.y) * (B.x - O.x);
}
vector<Point> convex_hull(vector<Point>& points) {
int n = points.size(), k = 0;
vector<Point> hull(2 * n);
sort(points.begin(), points.end());
for (int i = 0; i < n; i++) {
while (k >= 2 && cross(hull[k - 2], hull[k - 1], points[i]) <= 0) k--;
hull[k++] = points[i];
}
for (int i = n - 2, t = k + 1; i >= 0; i--) {
while (k >= t && cross(hull[k - 2], hull[k - 1], points[i]) <= 0) k--;
hull[k++] = points[i];
}
hull.resize(k - 1);
return hull;
}
double polygon_area(vector<Point>& points) {
int n = points.size();
double area = 0.0;
for (int i = 1; i < n - 1; i++) {
double x1 = points[i].x - points[0].x;
double y1 = points[i].y - points[0].y;
double x2 = points[i + 1].x - points[0].x;
double y2 = points[i + 1].y - points[0].y;
area += x1 * y2 - x2 * y1;
}
return abs(area) / 2.0;
}
vector<Point> intersection(vector<Point>& a, vector<Point>& b) {
vector<Point> res;
for (int i = 0; i < a.size(); i++) {
Point p1 = a[i];
Point p2 = a[(i + 1) % a.size()];
for (int j = 0; j < b.size(); j++) {
Point q1 = b[j];
Point q2 = b[(j + 1) % b.size()];
double d1 = cross(p1, p2, q1);
double d2 = cross(p1, p2, q2);
double d3 = cross(q1, q2, p1);
double d4 = cross(q1, q2, p2);
if ((d1 > 0 && d2 < 0 || d1 < 0 && d2 > 0) &&
(d3 > 0 && d4 < 0 || d3 < 0 && d4 > 0)) {
double t = d1 / (d1 - d2);
double x = q1.x + t * (q2.x - q1.x);
double y = q1.y + t * (q2.y - q1.y);
res.push_back(Point(x, y));
}
}
}
return convex_hull(res);
}
int main() {
vector<Point> a = { {0.0, 0.0}, {0.0, 1.0}, {1.0, 0.0}, {1.0, 1.0} };
vector<Point> b = { {0.5, 0.5}, {0.5, 1.5}, {1.5, 0.5}, {1.5, 1.5} };
vector<Point> hull_a = convex_hull(a);
vector<Point> hull_b = convex_hull(b);
double area_a = polygon_area(hull_a);
double area_b = polygon_area(hull_b);
vector<Point> inter = intersection(hull_a, hull_b);
double area_inter = polygon_area(inter);
cout << "Convex hull of A: ";
for (auto& p : hull_a) {
cout << "(" << p.x << ", " << p.y << ") ";
}
cout << endl;
cout << "Convex hull of B: ";
for (auto& p : hull_b) {
cout << "(" << p.x << ", " << p.y << ") ";
}
cout << endl;
cout << "Intersection of A and B: ";
for (auto& p : inter) {
cout << "(" << p.x << ", " << p.y << ") ";
}
cout << endl;
cout << "Area of A: " << area_a << endl;
cout << "Area of B: " << area_b << endl;
cout << "Area of intersection: " << area_inter << endl;
return 0;
}
```
在这个例子中,我们使用了四个点构成两组点云数据A和B,然后使用convex_hull函数求出了它们的凸包,接着使用polygon_area函数计算凸包面积,最后使用intersection函数求出两组点云数据的交集,并使用polygon_area函数计算交集面积。
相关推荐
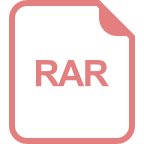














