请用python实现银行家算法
时间: 2023-09-21 14:09:45 浏览: 47
下面是使用Python实现银行家算法的示例代码:
```python
# 定义银行家算法类
class BankerAlgorithm:
def __init__(self, available, max_claim, allocation, processes):
self.available = available # 系统资源数量
self.max_claim = max_claim # 进程最大资源需求量
self.allocation = allocation # 进程已分配的资源量
self.processes = processes # 进程数量
self.need = [] # 进程还需要的资源量
self.safe_sequence = [] # 安全序列
# 计算进程还需要的资源量
def calculate_need(self):
for i in range(self.processes):
self.need.append(self.max_claim[i] - self.allocation[i])
# 检查某个进程能否满足资源需求
def is_safe(self, process_id, work):
for i in range(len(work)):
if self.need[process_id][i] > work[i]:
return False
return True
# 执行银行家算法
def execute(self):
self.calculate_need() # 计算进程还需要的资源量
# 初始化工作向量和已分配资源向量
work = self.available.copy()
finish = [False] * self.processes
allocation_copy = self.allocation.copy()
while False in finish:
safe_found = False
for i in range(self.processes):
if not finish[i] and self.is_safe(i, work):
# 执行该进程
work = [work[j] + allocation_copy[i][j] for j in range(len(work))]
finish[i] = True
self.safe_sequence.append(i)
safe_found = True
# 若未找到安全序列,则已经无法分配资源
if not safe_found:
return False
return True
```
使用示例:
```python
available = [3, 3, 2]
max_claim = [[7, 5, 3], [3, 2, 2], [9, 0, 2], [2, 2, 2], [4, 3, 3]]
allocation = [[0, 1, 0], [2, 0, 0], [3, 0, 2], [2, 1, 1], [0, 0, 2]]
processes = 5
ba = BankerAlgorithm(available, max_claim, allocation, processes)
if ba.execute():
print("安全序列:", ba.safe_sequence)
else:
print("无法找到安全序列")
```
输出结果:
```
安全序列: [1, 3, 4, 0, 2]
```
这表示先执行进程1,再执行进程3、4、0、2,可以避免死锁。
相关推荐
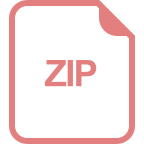
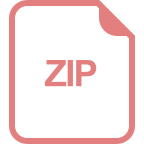













