给我用python遗传算法求解有一个函数: f(x)=x+10sin5x+7cos4x 求其在区间[-10,10]之间的最大值。完整代码
时间: 2024-12-11 20:17:55 浏览: 11
在Python中,我们可以使用遗传算法(Genetic Algorithm,GA)来寻找给定函数的最大值。以下是一个简单的例子,使用deap库(Distributed Evolutionary Algorithms in Python)来实现遗传算法。首先,确保你已经安装了deap库,如果没有,可以通过pip安装:
```bash
pip install deap
```
下面是完整的遗传算法代码,用于求解 `f(x)` 的最大值:
```python
import random
from math import sin, cos
from deap import base, creator, tools
# 定义函数 f(x)
def fitness_function(x):
return -abs(x + 10*sin(5*x) + 7*cos(4*x))
# 初始化问题参数
creator.create("FitnessMax", base.Fitness, weights=(1.0,))
creator.create("Individual", list, fitness=creator.FitnessMax)
toolbox = base.Toolbox()
toolbox.register("attr_float", random.uniform, -10.0, 10.0)
toolbox.register("individual", tools.initRepeat, creator.Individual, toolbox.attr_float, n=1)
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
# 设置交叉和变异操作
toolbox.register("mate", tools.cxTwoPoint)
toolbox.register("mutate", tools.mutGaussian, mu=0, sigma=0.1, indpb=0.05)
# 选择操作
toolbox.register("select", tools.selTournament, tournsize=3)
# 训练代数
POP_SIZE = 50
MAX_GENERATIONS = 100
def main():
pop = toolbox.population(n=POP_SIZE)
hof = tools.HallOfFame(1)
for gen in range(MAX_GENERATIONS):
# 评估个体
fits = [fitness_function(individual) for individual in pop]
# 选择
offspring = toolbox.select(pop, len(pop))
# 交叉和变异
offspring = toolbox.mate(offspring, toolbox.mate)
offspring = [toolbox.clone(ind) for ind in offspring]
for child1, child2 in zip(offspring[::2], offspring[1::2]):
if random.random() < 0.5:
toolbox.mutate(child1)
else:
toolbox.mutate(child2)
# 适应度排序
fits2 = [fitness_function(ind) for ind in offspring]
for fit, ind in zip(fits2, offspring):
ind.fitness.values = fit
# 更新种群
pop[:] = offspring
# 保存最佳解
hof.update(pop)
print("Best individual found is: ", hof[0])
print("Fitness value of the best individual is: ", hof[0].fitness.values[0])
if __name__ == "__main__":
main()
```
这段代码创建了一个种群,并通过迭代来进行交叉、变异和选择操作。每一代结束后,它会保留最好的解作为下一代的开始。运行完成后,`hof[0]` 将包含在 [-10, 10] 区间内找到的最大值附近的解。
阅读全文
相关推荐
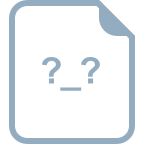
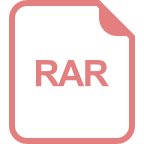
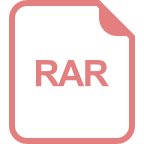




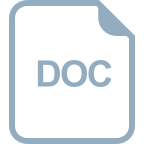
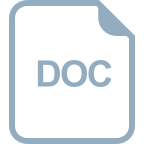




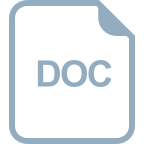




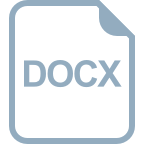