c++如何实现一元多项式的相加和相乘
时间: 2024-09-29 08:05:46 浏览: 58
在C++中实现一元多项式(Polynomial)的相加和相乘通常会涉及到结构体或者类来存储系数和次数,这里提供一个简单的例子:
首先,我们可以定义一个`Term`结构体,表示一次项(系数-指数的形式):
```cpp
struct Term {
int coefficient;
int exponent;
};
```
然后,创建一个`Polynomial`类,包含一个`std::vector<Term>`来存储多项式的各个项:
```cpp
class Polynomial {
private:
std::vector<Term> terms;
public:
// 添加项的方法
void addTerm(int coefficient, int exponent) {
terms.push_back({coefficient, exponent});
}
// 相加两个多项式
Polynomial operator+(const Polynomial& other) const {
Polynomial result;
for (const auto& term : terms) {
for (const auto& otherTerm : other.terms) {
if (term.exponent == otherTerm.exponent) {
result.addTerm(term.coefficient + otherTerm.coefficient, term.exponent);
break; // 同次幂只保留一个
}
}
}
return result;
}
// 相乘两个多项式(假设没有合并重复项)
Polynomial operator*(const Polynomial& other) const {
Polynomial result;
for (const auto& term : terms) {
for (const auto& otherTerm : other.terms) {
result.addTerm(term.coefficient * otherTerm.coefficient, term.exponent + otherTerm.exponent);
}
}
return result;
}
};
```
这样,你可以创建两个`Polynomial`对象并进行相加和相乘操作:
```cpp
int main() {
Polynomial p1 = {4, 2}; // 4x^2
Polynomial p2 = {3, 0}; // 3
Polynomial sum = p1 + p2; // 7x^2 + 3
Polynomial product = p1 * p2; // 12x^2 + 12x
// 输出结果...
}
```
阅读全文
相关推荐
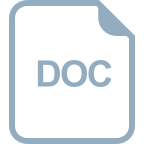
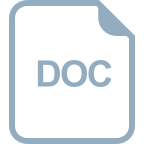
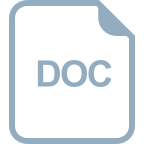

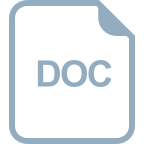

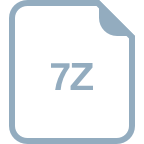
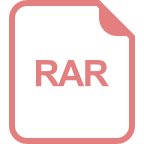


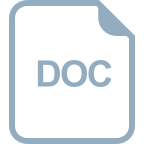
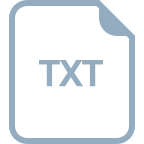
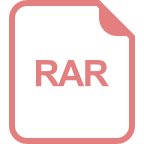
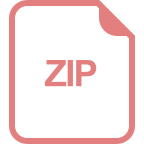
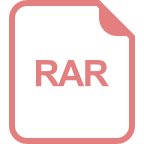