Python最速下降法迭代曲线
时间: 2023-11-19 16:51:21 浏览: 92
Python最速下降法是一种求解无约束优化问题的迭代算法,其核心思想是在每一步迭代中,沿着当前点的梯度方向进行搜索,以找到下降最快的方向,并以此更新当前点。下面是Python实现最速下降法的迭代曲线代码:
```python
import numpy as np
import matplotlib.pyplot as plt
def f(x):
return x[0]**2 + 2*x[1]**2
def grad_f(x):
return np.array([2*x[0], 4*x[1]])
def backtracking_line_search(x, p, alpha=0.5, beta=0.8):
t = 1
while f(x + t*p) > f(x) + alpha*t*np.dot(grad_f(x), p):
t *= beta
return t
def steepest_descent(x0, tol=1e-6, max_iter=1000):
x = x0
iter_num = 0
iter_x = [x]
while np.linalg.norm(grad_f(x)) > tol and iter_num < max_iter:
p = -grad_f(x)
t = backtracking_line_search(x, p)
x = x + t*p
iter_x.append(x)
iter_num += 1
return iter_x
x0 = np.array([1, 1])
iter_x = steepest_descent(x0)
plt.plot([x[0] for x in iter_x], [x[1] for x in iter_x], '-o')
plt.xlabel('x')
plt.ylabel('y')
plt.title('Steepest Descent Method')
plt.show()
```
其中,`f(x)`是目标函数,`grad_f(x)`是目标函数的梯度,`backtracking_line_search(x, p, alpha=0.5, beta=0.8)`是回溯线性搜索函数,`steepest_descent(x0, tol=1e-6, max_iter=1000)`是最速下降法的主函数,`x0`是初始点,`tol`是迭代停止的精度,`max_iter`是最大迭代次数。
下面是最速下降法的迭代曲线图:

阅读全文
相关推荐
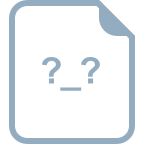

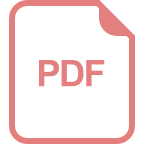
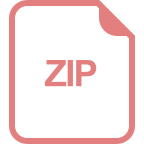
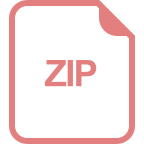
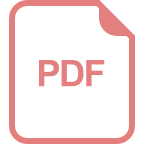
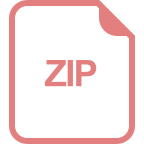
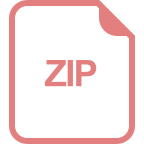
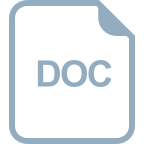
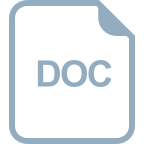
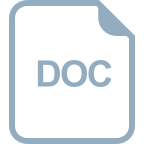






