帮我优化这段代码:def readCSVFile(demand_file,depot_file,model): with open(demand_file,'r') as f: demand_reader=csv.demand_reader 中行的 DictReader(f):需求 = 需求() demand.id = int(row['id']) demand.x_coord = float(row['x_coord']) demand.y_coord = float(row['y_coord']) demand.demand = float(row['demand']) demand.start_time=float(row['start_time']) demand.end_time=float(row['end_time']) demand.service_time=float(row['service_time']) model.demand_dict[demand.id] = 需求 model.demand_id_list.append(demand.id) model.number_of_demands=len(model.demand_id_list) with open(depot_file, 'r') 作为 f: depot_reader = csv。depot_reader 中行的字典阅读器(f):车辆 = 车辆() vehicle.depot_id = 行['depot_id'] vehicle.x_coord = float(row['x_coord']) vehicle.y_coord = float(row['y_coord']) vehicle.type = row['vehicle_type'] vehicle.capacity=float(row['vehicle_capacity']) vehicle.free_speed=float(row['vehicle_speed']) vehicle.numbers=float(row['number_of_vehicle']) vehicle.fixed_cost=float(row['fixed_cost']) vehicle.variable_cost=float(row['variable_cost']) vehicle.start_time=float(row['start_time']) vehicle.end_time=float(row['end_time']) model.vehicle_dict[vehicle.type] = Vehicle model.vehicle_type_list.append(vehicle.type)
时间: 2024-02-23 14:01:27 浏览: 89
可以考虑使用 Pandas 库来读取 CSV 文件,这样可以更加高效地读取和处理数据。下面是使用 Pandas 的示例代码:
```python
import pandas as pd
def readCSVFile(demand_file, depot_file, model):
demand_df = pd.read_csv(demand_file)
demand_df['id'] = demand_df['id'].astype(int)
demand_df['x_coord'] = demand_df['x_coord'].astype(float)
demand_df['y_coord'] = demand_df['y_coord'].astype(float)
demand_df['demand'] = demand_df['demand'].astype(float)
demand_df['start_time'] = demand_df['start_time'].astype(float)
demand_df['end_time'] = demand_df['end_time'].astype(float)
demand_df['service_time'] = demand_df['service_time'].astype(float)
model.demand_dict = demand_df.set_index('id').to_dict('index')
model.demand_id_list = demand_df['id'].tolist()
model.number_of_demands = len(model.demand_id_list)
depot_df = pd.read_csv(depot_file)
depot_df['x_coord'] = depot_df['x_coord'].astype(float)
depot_df['y_coord'] = depot_df['y_coord'].astype(float)
depot_df['capacity'] = depot_df['vehicle_capacity'].astype(float)
depot_df['free_speed'] = depot_df['vehicle_speed'].astype(float)
depot_df['numbers'] = depot_df['number_of_vehicle'].astype(float)
depot_df['fixed_cost'] = depot_df['fixed_cost'].astype(float)
depot_df['variable_cost'] = depot_df['variable_cost'].astype(float)
depot_df['start_time'] = depot_df['start_time'].astype(float)
depot_df['end_time'] = depot_df['end_time'].astype(float)
model.vehicle_dict = depot_df.set_index('vehicle_type').to_dict('index')
model.vehicle_type_list = depot_df['vehicle_type'].tolist()
```
这里使用了 Pandas 的 `read_csv` 方法来读取 CSV 文件,并且对列进行了类型转换。读取的数据可以通过 `set_index` 方法设置索引,然后转换为字典。最后将需要的数据存储到 `model` 对象中。
阅读全文
相关推荐
















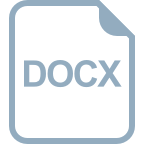