用c++写一个指数函数计算器
时间: 2023-11-24 08:08:01 浏览: 109
以下是一个用C++编写的指数函数计算器的示例代码:
```cpp
#include <iostream>
#include <vector>
using namespace std;
// 定义多项式结构体
struct Poly {
int coef; // 系数
int exp; // 指数
};
// 求多项式的导数
vector<Poly> derivative(vector<Poly>& poly) {
vector<Poly> res;
for (int i = 0; i < poly.size(); i++) {
if (poly[i].exp == 0) { // 常数项求导等于0,跳过
continue;
}
Poly temp;
temp.coef = poly[i].coef * poly[i].exp;
temp.exp = poly[i].exp - 1;
res.push_back(temp);
}
return res;
}
// 输出多项式
void display(vector<Poly>& poly) {
for (int i = 0; i < poly.size(); i++) {
if (poly[i].coef == 0) { // 系数为0,跳过
continue;
}
if (i != 0 && poly[i].coef > 0) { // 非首项且系数为正数,输出加号
cout << "+";
}
if (poly[i].coef == -1 && poly[i].exp != 0) { // 系数为-1,只输出负号
cout << "-";
} else if (poly[i].coef != 1 || poly[i].exp == 0) { // 系数不为1或指数为0,输出系数
cout << poly[i].coef;
}
if (poly[i].exp != 0) { // 指数不为0,输出x
cout << "x";
}
if (poly[i].exp > 1) { // 指数大于1,输出指数
cout << "^" << poly[i].exp;
}
}
cout << endl;
}
int main() {
vector<Poly> poly = {{2, 3}, {-1, 2}, {3, 1}, {4, 0}}; // 多项式2x^3-x^2+3x+4
vector<Poly> res = derivative(poly); // 求导
display(res); // 输出导数
return 0;
}
```
阅读全文
相关推荐



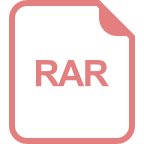
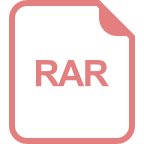




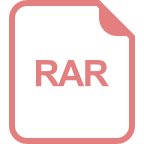





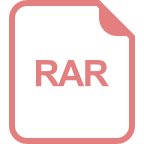


