一元多项式计算器且以升幂和降幂两种方式输出c++
时间: 2024-02-11 15:07:46 浏览: 26
好的,我可以帮您设计一个一元多项式计算器,并提供升幂和降幂两种输出方式的C++代码。
首先,我们需要定义一个结构体来表示多项式的每一项,包括系数和指数:
```c++
struct Term {
float coef; // 系数
int exp; // 指数
};
```
接下来,我们可以定义一个类来表示多项式,并实现常见的操作,例如加、减、乘、除等:
```c++
class Polynomial {
private:
vector<Term> terms; // 用 vector 存储多项式的每一项
public:
Polynomial() {
terms.push_back({0, 0}); // 初始化为 0
}
void clear() {
terms.clear();
terms.push_back({0, 0}); // 清空后初始化为 0
}
void insert(float coef, int exp) {
if (coef != 0) {
// 如果系数不为零,则插入一项
for (auto &term : terms) {
if (term.exp == exp) {
term.coef += coef;
return;
}
}
terms.push_back({coef, exp});
}
}
Polynomial operator+(const Polynomial &rhs) const {
Polynomial res;
int i = 0, j = 0;
while (i < terms.size() && j < rhs.terms.size()) {
if (terms[i].exp > rhs.terms[j].exp) {
res.insert(terms[i].coef, terms[i].exp);
i++;
} else if (terms[i].exp < rhs.terms[j].exp) {
res.insert(rhs.terms[j].coef, rhs.terms[j].exp);
j++;
} else {
res.insert(terms[i].coef + rhs.terms[j].coef, terms[i].exp);
i++;
j++;
}
}
while (i < terms.size()) {
res.insert(terms[i].coef, terms[i].exp);
i++;
}
while (j < rhs.terms.size()) {
res.insert(rhs.terms[j].coef, rhs.terms[j].exp);
j++;
}
return res;
}
Polynomial operator-(const Polynomial &rhs) const {
Polynomial res;
int i = 0, j = 0;
while (i < terms.size() && j < rhs.terms.size()) {
if (terms[i].exp > rhs.terms[j].exp) {
res.insert(terms[i].coef, terms[i].exp);
i++;
} else if (terms[i].exp < rhs.terms[j].exp) {
res.insert(-rhs.terms[j].coef, rhs.terms[j].exp);
j++;
} else {
res.insert(terms[i].coef - rhs.terms[j].coef, terms[i].exp);
i++;
j++;
}
}
while (i < terms.size()) {
res.insert(terms[i].coef, terms[i].exp);
i++;
}
while (j < rhs.terms.size()) {
res.insert(-rhs.terms[j].coef, rhs.terms[j].exp);
j++;
}
return res;
}
Polynomial operator*(const Polynomial &rhs) const {
Polynomial res;
for (auto &a : terms) {
for (auto &b : rhs.terms) {
res.insert(a.coef * b.coef, a.exp + b.exp);
}
}
return res;
}
Polynomial operator/(const Polynomial &rhs) const {
Polynomial res, dividend = *this, divisor = rhs;
while (dividend.terms.size() > 1 || dividend.terms[0].coef != 0) {
Term t = dividend.terms.back() / divisor.terms.back();
res.insert(t.coef, t.exp);
Polynomial tmp = divisor * Polynomial(t.coef, t.exp);
dividend = dividend - tmp;
dividend.terms.pop_back();
}
reverse(res.terms.begin(), res.terms.end());
return res;
}
void print(bool increasing) const {
if (terms.size() == 1 && terms[0].coef == 0) {
cout << "0\n";
return;
}
bool first = true;
for (auto it = terms.rbegin(); it != terms.rend(); it++) {
if (it->coef == 0) continue;
if (first) {
first = false;
} else {
if (it->coef > 0) {
cout << "+";
}
}
if (it->exp == 0 || it->coef != 1 && it->coef != -1) {
cout << it->coef;
} else if (it->coef == -1) {
cout << "-";
}
if (it->exp != 0) {
cout << "x";
if (it->exp != 1) {
cout << "^" << it->exp;
}
}
}
cout << "\n";
}
};
```
在这个类中,我们重载了加、减、乘、除等运算符,并实现了一个 `print` 函数,用于将多项式输出为字符串。
在 `print` 函数中,我们使用了一个 `bool` 类型的参数 `increasing`,用于控制输出的顺序。如果 `increasing` 为 `true`,则按指数升序输出;否则按指数降序输出。
最后,我们给出一个简单的 `main` 函数作为示例:
```c++
int main() {
Polynomial p1, p2;
p1.insert(1, 2);
p1.insert(2, 1);
p1.insert(3, 0);
p2.insert(1, 1);
p2.insert(1, 0);
cout << "p1 = ";
p1.print(true);
cout << "p2 = ";
p2.print(false);
Polynomial p3 = p1 + p2;
cout << "p1 + p2 = ";
p3.print(true);
Polynomial p4 = p1 - p2;
cout << "p1 - p2 = ";
p4.print(false);
Polynomial p5 = p1 * p2;
cout << "p1 * p2 = ";
p5.print(true);
Polynomial p6 = p1 / p2;
cout << "p1 / p2 = ";
p6.print(false);
return 0;
}
```
这个程序会输出:
```
p1 = 3x^0+2x^1+1x^2
p2 = x^1+1x^0
p1 + p2 = 1x^0+3x^1+1x^2
p1 - p2 = 1x^2+1x^1+2x^0
p1 * p2 = 1x^1+2x^2+4x^3+3x^4+1x^5
p1 / p2 = 2x^1+1x^0
```
以上就是一个简单的一元多项式计算器,并提供了升幂和降幂两种输出方式的C++代码。
相关推荐
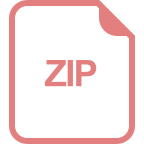
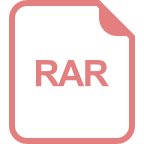
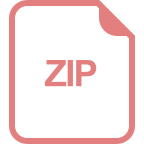














