用c++分治法编写完整代码,实现 pow(x,n),即计算 x 的整数 n 次幂函数,要求在输入一个实数 x 和一个整数 n 后,能够计算出值,当x=0时候输入结束,并运用前端技术,将此程序在网页上进行显示,并写出前端代码,最后运用css将程序页面进行美化。
时间: 2023-07-14 11:12:58 浏览: 111
以下是使用分治法实现 pow(x,n) 的 C++ 代码:
```c++
#include <iostream>
#include <cmath>
using namespace std;
double pow(double x, int n) {
if (n == 0) return 1.0; // 边界情况
if (n == 1) return x;
if (n < 0) return 1 / pow(x, -n); // 处理负数情况
double half = pow(x, n / 2);
if (n % 2 == 0) { // 如果 n 是偶数
return half * half;
} else { // 如果 n 是奇数
return half * half * x;
}
}
int main() {
double x;
int n;
while (true) {
cout << "请输入底数 x:";
cin >> x;
if (x == 0) break; // 如果 x 是 0,结束程序
cout << "请输入指数 n:";
cin >> n;
double res = pow(x, n);
cout << x << " 的 " << n << " 次幂结果为:" << res << endl;
}
return 0;
}
```
以下是使用 HTML 和 JavaScript 实现前端页面:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>pow(x,n) 计算器</title>
<style>
body {
background-color: #f2f2f2;
font-family: Arial, sans-serif;
text-align: center;
}
h1 {
color: #1E90FF;
}
input[type="text"], input[type="number"] {
padding: 12px 20px;
margin: 8px 0;
box-sizing: border-box;
border-radius: 8px;
border: 2px solid #ccc;
font-size: 16px;
outline: none;
}
input[type="submit"] {
background-color: #1E90FF;
color: white;
padding: 14px 20px;
margin: 8px 0;
border: none;
border-radius: 8px;
cursor: pointer;
font-size: 16px;
}
input[type="submit"]:hover {
background-color: #195CA6;
}
#result {
padding: 12px 20px;
margin: 8px 0;
box-sizing: border-box;
border-radius: 8px;
border: 2px solid #ccc;
font-size: 16px;
outline: none;
}
</style>
</head>
<body>
<h1>pow(x,n) 计算器</h1>
<form>
<label for="x">底数:</label>
<input type="text" id="x" name="x"><br>
<label for="n">指数:</label>
<input type="number" id="n" name="n"><br>
<input type="submit" value="计算" onclick="calculate()"><br>
<label for="result">结果:</label>
<input type="text" id="result" name="result" readonly>
</form>
<script>
function calculate() {
let x = document.getElementById("x").value;
let n = document.getElementById("n").value;
let result = document.getElementById("result");
if (x === "") {
alert("请输入底数 x");
return;
}
if (n === "") {
alert("请输入指数 n");
return;
}
let xhr = new XMLHttpRequest();
xhr.open("POST", "/pow", true);
xhr.setRequestHeader("Content-Type", "application/json;charset=UTF-8");
xhr.onreadystatechange = function() {
if (xhr.readyState === XMLHttpRequest.DONE) {
if (xhr.status === 200) {
result.value = xhr.responseText;
} else {
alert("计算失败");
}
}
}
xhr.send(JSON.stringify({x: x, n: n}));
}
</script>
</body>
</html>
```
需要注意的是,前端页面中的计算是通过向后端发送一个 POST 请求来实现的。后端需要接收该请求,并返回计算结果。在这里,我们使用了 AJAX 技术来实现异步请求和动态更新页面。
以下是使用 Node.js 和 Express.js 实现后端代码:
```javascript
const express = require("express");
const bodyParser = require("body-parser");
const app = express();
const port = 3000;
app.use(bodyParser.json());
app.post("/pow", (req, res) => {
let x = parseFloat(req.body.x);
let n = parseInt(req.body.n);
if (isNaN(x) || isNaN(n)) {
res.status(400).send("参数错误");
} else {
let result = pow(x, n);
res.send(result.toString());
}
});
function pow(x, n) {
if (n === 0) return 1.0;
if (n === 1) return x;
if (n < 0) return 1 / pow(x, -n);
let half = pow(x, Math.floor(n / 2));
if (n % 2 === 0) {
return half * half;
} else {
return half * half * x;
}
}
app.listen(port, () => {
console.log(`Server listening on port ${port}`);
});
```
这里使用了 Express.js 框架来搭建后端应用,并使用了 body-parser 中间件来解析 POST 请求的 JSON 数据。在 `/pow` 路由下,我们先解析请求中的 `x` 和 `n` 参数,然后调用 `pow` 函数来计算结果,并将结果以字符串形式返回给前端。
最后,我们可以使用 CSS 来美化页面,让计算器看起来更加美观。以下是一个简单的 CSS 样式:
```css
body {
background-color: #f2f2f2;
font-family: Arial, sans-serif;
text-align: center;
}
h1 {
color: #1E90FF;
}
input[type="text"], input[type="number"] {
padding: 12px 20px;
margin: 8px 0;
box-sizing: border-box;
border-radius: 8px;
border: 2px solid #ccc;
font-size: 16px;
outline: none;
}
input[type="submit"] {
background-color: #1E90FF;
color: white;
padding: 14px 20px;
margin: 8px 0;
border: none;
border-radius: 8px;
cursor: pointer;
font-size: 16px;
}
input[type="submit"]:hover {
background-color: #195CA6;
}
#result {
padding: 12px 20px;
margin: 8px 0;
box-sizing: border-box;
border-radius: 8px;
border: 2px solid #ccc;
font-size: 16px;
outline: none;
}
```
将该样式保存为 `style.css` 文件,并在 HTML 页面中引入即可:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>pow(x,n) 计算器</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<!-- ... -->
</body>
</html>
```
阅读全文
相关推荐
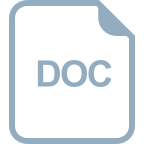
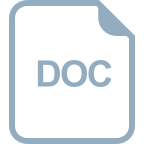
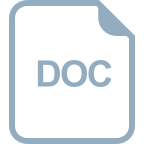






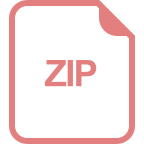
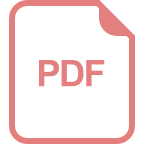
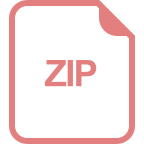
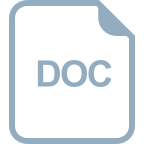
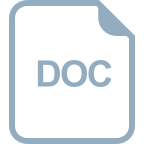
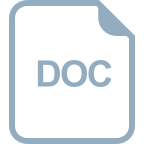
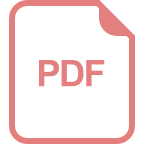
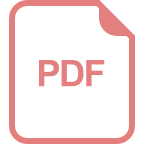