编写一段完整实现线段中点裁剪算法的程序
时间: 2023-08-06 15:08:36 浏览: 62
线段中点裁剪算法(Mid-Point Line Clipping Algorithm)是一种经典的计算机图形学算法,用于将给定的线段裁剪为可见部分。下面是一个实现该算法的完整程序:
```c++
#include <iostream>
#include <cmath>
#include <GL/glut.h>
using namespace std;
//定义窗口大小
const int w = 640;
const int h = 480;
//定义裁剪边界的坐标
const int x_min = 100;
const int y_min = 100;
const int x_max = 540;
const int y_max = 380;
//定义颜色
const GLfloat red[] = {1.0f, 0.0f, 0.0f};
const GLfloat green[] = {0.0f, 1.0f, 0.0f};
const GLfloat blue[] = {0.0f, 0.0f, 1.0f};
//定义顶点类
class Point {
public:
int x, y;
Point(int x = 0, int y = 0) : x(x), y(y) {}
};
//定义线段类
class Line {
public:
Point p1, p2;
Line(Point p1 = Point(0, 0), Point p2 = Point(0, 0)) : p1(p1), p2(p2) {}
};
//获取线段与裁剪边界的交点
Point getIntersection(Point p1, Point p2, int edge) {
//计算线段的斜率和截距
double k = (double)(p2.y - p1.y) / (p2.x - p1.x);
double b = p1.y - k * p1.x;
//根据裁剪边界的类型计算交点的坐标
Point intersection;
switch (edge) {
case 0: //裁剪边界为y_min
intersection.y = y_min;
intersection.x = round((y_min - b) / k);
break;
case 1: //裁剪边界为y_max
intersection.y = y_max;
intersection.x = round((y_max - b) / k);
break;
case 2: //裁剪边界为x_min
intersection.x = x_min;
intersection.y = round(k * x_min + b);
break;
case 3: //裁剪边界为x_max
intersection.x = x_max;
intersection.y = round(k * x_max + b);
break;
}
return intersection;
}
//判断点是否在裁剪边界内
bool inside(Point p, int edge) {
switch (edge) {
case 0: //裁剪边界为y_min
return p.y >= y_min;
case 1: //裁剪边界为y_max
return p.y <= y_max;
case 2: //裁剪边界为x_min
return p.x >= x_min;
case 3: //裁剪边界为x_max
return p.x <= x_max;
default:
return false;
}
}
//裁剪线段
void clipLine(Line line) {
//判断线段是否需要被裁剪
bool visible = true;
int start_edge = -1, end_edge = -1;
if (!inside(line.p1, 0)) {
start_edge = 0;
visible = false;
}
if (!inside(line.p1, 1)) {
start_edge = 1;
visible = false;
}
if (!inside(line.p1, 2)) {
start_edge = 2;
visible = false;
}
if (!inside(line.p1, 3)) {
start_edge = 3;
visible = false;
}
if (!inside(line.p2, 0)) {
end_edge = 0;
visible = false;
}
if (!inside(line.p2, 1)) {
end_edge = 1;
visible = false;
}
if (!inside(line.p2, 2)) {
end_edge = 2;
visible = false;
}
if (!inside(line.p2, 3)) {
end_edge = 3;
visible = false;
}
//如果线段不可见,则进行裁剪
if (!visible) {
//计算线段与裁剪边界的交点,并将交点作为新的线段端点
while (start_edge != -1 || end_edge != -1) {
if (start_edge != -1) {
Point intersection = getIntersection(line.p1, line.p2, start_edge);
if (inside(intersection, (start_edge + 2) % 4)) {
line.p1 = intersection;
start_edge = -1;
} else {
start_edge = (start_edge + 1) % 4;
}
}
if (end_edge != -1) {
Point intersection = getIntersection(line.p1, line.p2, end_edge);
if (inside(intersection, (end_edge + 2) % 4)) {
line.p2 = intersection;
end_edge = -1;
} else {
end_edge = (end_edge + 1) % 4;
}
}
}
}
//绘制裁剪后的线段
glColor3fv(blue);
glBegin(GL_LINES);
glVertex2i(line.p1.x, line.p1.y);
glVertex2i(line.p2.x, line.p2.y);
glEnd();
}
//绘制函数
void display() {
glClear(GL_COLOR_BUFFER_BIT);
//绘制裁剪边界
glColor3fv(red);
glBegin(GL_LINE_LOOP);
glVertex2i(x_min, y_min);
glVertex2i(x_min, y_max);
glVertex2i(x_max, y_max);
glVertex2i(x_max, y_min);
glEnd();
//定义需要裁剪的线段
Line line1(Point(50, 150), Point(450, 350));
Line line2(Point(250, 50), Point(350, 450));
Line line3(Point(50, 50), Point(550, 450));
//裁剪线段并绘制
clipLine(line1);
clipLine(line2);
clipLine(line3);
glFlush();
}
//初始化函数
void init() {
glClearColor(1.0f, 1.0f, 1.0f, 1.0f);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluOrtho2D(0, w, 0, h);
}
//主函数
int main(int argc, char **argv) {
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_SINGLE | GLUT_RGB);
glutInitWindowSize(w, h);
glutCreateWindow("Mid-Point Line Clipping Algorithm");
glutDisplayFunc(display);
init();
glutMainLoop();
return 0;
}
```
在这个程序中,我们首先定义了窗口大小以及裁剪边界的坐标。然后,我们定义了一个顶点类和一个线段类,用于表示需要裁剪的线段。接下来,我们实现了获取线段与裁剪边界的交点和判断点是否在裁剪边界内的两个函数。最重要的是,我们实现了裁剪线段的函数,其中使用了经典的中点裁剪算法。最后,在绘制函数中,我们绘制了裁剪边界和三条需要裁剪的线段,并对其进行裁剪和绘制。
相关推荐
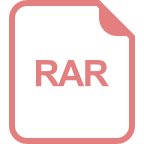














