c++用栈实现复杂表达式求值代码
时间: 2023-10-30 08:08:45 浏览: 92
以下是使用栈实现复杂表达式求值的C++代码:
```c++
#include <iostream>
#include <stack>
#include <string>
#include <cstring>
#include <cmath>
using namespace std;
// 优先级比较函数
int cmp(char op1, char op2) {
if (op1 == '(' || op2 == '(') {
return -1;
}
if ((op1 == '*' || op1 == '/') && (op2 == '+' || op2 == '-')) {
return -1;
} else {
return 1;
}
}
// 计算函数
double calc(double num1, double num2, char op) {
switch (op) {
case '+': return num1 + num2;
case '-': return num1 - num2;
case '*': return num1 * num2;
case '/': return num1 / num2;
case '^': return pow(num1, num2);
default: return 0;
}
}
// 将中缀表达式转成后缀表达式
string infixToPostfix(string infix) {
stack<char> s;
string postfix;
for (int i = 0; i < infix.length(); i++) {
char c = infix[i];
if (isdigit(c) || c == '.') { // 数字和小数点直接输出
postfix += c;
continue;
}
postfix += ' '; // 操作符之间加空格
while (!s.empty() && cmp(s.top(), c) == 1) { // 栈顶元素优先级大于等于当前操作符
postfix += s.top();
s.pop();
postfix += ' ';
}
if (c == ')') { // 如果是右括号,弹出直到左括号
while (!s.empty() && s.top() != '(') {
postfix += s.top();
s.pop();
postfix += ' ';
}
s.pop();
} else { // 其他情况直接入栈
s.push(c);
}
}
while (!s.empty()) {
postfix += ' ';
postfix += s.top();
s.pop();
}
return postfix;
}
// 计算后缀表达式
double calcPostfix(string postfix) {
stack<double> s;
char buf[100];
for (int i = 0; i < postfix.length(); i++) {
char c = postfix[i];
if (isdigit(c) || c == '.') { // 数字直接入栈
int j = i, k = 0;
while (isdigit(postfix[j]) || postfix[j] == '.') {
buf[k++] = postfix[j++];
}
buf[k] = '\0';
double num = atof(buf);
s.push(num);
i = j - 1;
} else if (c != ' ') { // 操作符弹出栈顶元素计算
double num2 = s.top();
s.pop();
double num1 = s.top();
s.pop();
s.push(calc(num1, num2, c));
}
}
return s.top();
}
int main() {
string infix;
cout << "请输入中缀表达式:";
getline(cin, infix);
string postfix = infixToPostfix(infix);
cout << "后缀表达式为:" << postfix << endl;
double result = calcPostfix(postfix);
cout << "计算结果为:" << result << endl;
return 0;
}
```
这个代码通过将中缀表达式转换为后缀表达式,然后使用栈计算后缀表达式的值来实现复杂表达式求值。其中,`cmp`函数用于比较两个操作符的优先级,`calc`函数用于计算两个数和一个操作符的结果,`infixToPostfix`函数用于将中缀表达式转换为后缀表达式,`calcPostfix`函数用于计算后缀表达式的值。
阅读全文
相关推荐

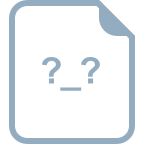
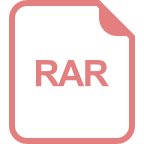
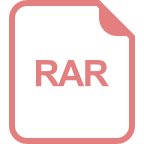
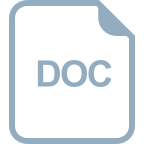
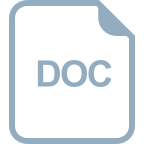
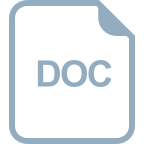
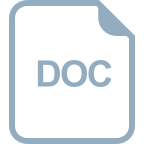


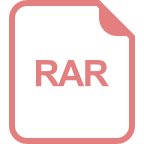
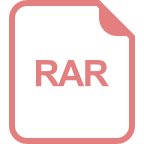
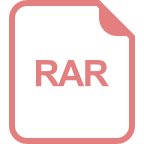
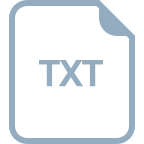
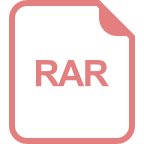
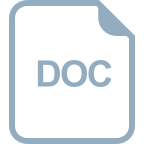