利用栈,进行表达式求值问题的解答,利用C++写出完整代码。注意:中缀运算符
时间: 2024-10-18 07:13:59 浏览: 14
在计算机科学中,解决中缀表达式求值的问题通常涉及到将中缀表达式转换成后缀(也称为逆波兰表示法,RPN),然后使用栈的数据结构来计算结果。这里是一个简单的C++代码示例,它使用了递归算法和两个栈:一个用于存储操作数,另一个用于存储操作符:
```cpp
#include <iostream>
#include <stack>
#include <string>
// 常量定义优先级
const int PRECEDENCE[] = {0, 0, 1, 2, 2, 3}; // 加减(0), 乘除(1, 2), 括号(3)
bool isOperator(const char op) {
return op == '+' || op == '-' || op == '*' || op == '/';
}
int getPrecedence(char op) {
for (int i = 0; i < sizeof(PRECEDENCE) / sizeof(PRECEDENCE[0]); ++i) {
if (op == PRECEDENCE[i]) return i;
}
return -1;
}
int applyOperator(int a, int b, char op) {
switch (op) {
case '+': return a + b;
case '-': return a - b;
case '*': return a * b;
case '/': return a / b;
default: assert(false && "Invalid operator");
}
}
std::pair<int, std::string> evaluateExpression(std::string expression) {
std::stack<int> values; // 存储操作数
std::stack<char> ops; // 存储操作符
int num = 0;
bool isNegative = false;
for (char c : expression) {
if (isdigit(c)) {
num = num * 10 + (c - '0');
if (!isNegative) values.push(num);
isNegative = false;
} else if (c == '-') {
isNegative = true;
} else if (isOperator(c)) {
while (!ops.empty() && getPrecedence(c) <= getPrecedence(ops.top())) {
values.push(applyOperator(values.top(), values.pop(), ops.top()));
ops.pop();
}
ops.push(c);
} else if (c == '(') {
ops.push(c);
} else if (c == ')') {
while (!ops.top() || ops.top() != '(') {
values.push(applyOperator(values.top(), values.pop(), ops.top()));
ops.pop();
}
ops.pop(); // pop the '('
}
}
while (!ops.empty()) {
values.push(applyOperator(values.top(), values.pop(), ops.top()));
ops.pop();
}
return {values.top(), isNegative ? -values.top() : values.top()};
}
int main() {
std::string expression = "3+5*2"; // 示例表达式
auto result = evaluateExpression(expression);
std::cout << "结果: " << result.first << ", 是否有负号: " << (result.second ? "有" : "无") << std::endl;
return 0;
}
```
这个程序会解析输入的中缀表达式并返回计算结果。请注意,这只是一个基本版本,实际应用中可能需要处理更复杂的情况,比如处理括号、处理错误输入等。
阅读全文
相关推荐
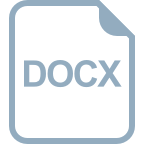
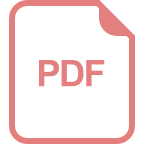
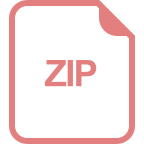
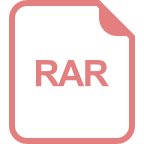
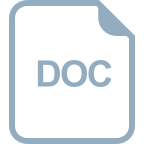


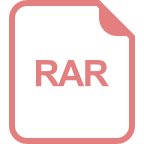
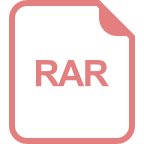
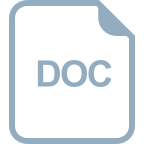
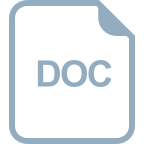
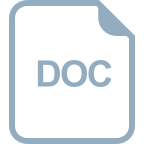
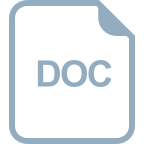
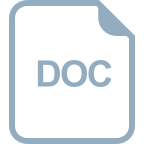




