使用C++进行编程,假设x和y是两个由单链表组成的字符串(其中每个结点只存储一个字符),请编写一个算法,找出x中第一个不在y中出现的字符。
时间: 2023-05-27 14:02:29 浏览: 70
思路:
先在y链表中建立一个哈希表,以字符为下标,记录字符出现的次数。然后遍历x链表,查找第一个不在哈希表中或者哈希表中次数为0的字符。
具体实现时,可以用一个数组freq来记录哈希表,freq[i]表示字符i出现的次数。
代码实现:
定义链表节点结构体:
```c
typedef struct ListNode {
char data;
struct ListNode* next;
} ListNode;
```
建立哈希表函数:
```c
void buildHash(ListNode* y, int* freq) {
while (y != NULL) {
freq[y->data]++;
y = y->next;
}
}
```
查找第一个不在哈希表中或者哈希表中次数为0的字符函数:
```c
char findFirst(ListNode* x, int* freq) {
while (x != NULL) {
if (freq[x->data] == 0) {
return x->data;
}
x = x->next;
}
return '\0'; // 找不到返回空字符
}
```
完整代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct ListNode {
char data;
struct ListNode* next;
} ListNode;
void buildHash(ListNode* y, int* freq) {
while (y != NULL) {
freq[y->data]++;
y = y->next;
}
}
char findFirst(ListNode* x, int* freq) {
while (x != NULL) {
if (freq[x->data] == 0) {
return x->data;
}
x = x->next;
}
return '\0'; // 找不到返回空字符
}
int main() {
// 构造x和y链表
ListNode* x = (ListNode*)malloc(sizeof(ListNode));
x->data = 'a';
x->next = (ListNode*)malloc(sizeof(ListNode));
x->next->data = 'b';
x->next->next = (ListNode*)malloc(sizeof(ListNode));
x->next->next->data = 'c';
x->next->next->next = NULL;
ListNode* y = (ListNode*)malloc(sizeof(ListNode));
y->data = 'c';
y->next = (ListNode*)malloc(sizeof(ListNode));
y->next->data = 'd';
y->next->next = NULL;
// 建立哈希表
int freq[256] = {0}; // 假设字符集为ASCII码,共256种字符
buildHash(y, freq);
// 查找第一个不在y中出现的字符
char ans = findFirst(x, freq);
printf("ans: %c\n", ans);
// 释放内存
ListNode* p = x;
while (p != NULL) {
ListNode* q = p->next;
free(p);
p = q;
}
p = y;
while (p != NULL) {
ListNode* q = p->next;
free(p);
p = q;
}
return 0;
}
```
相关推荐
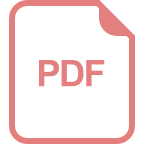
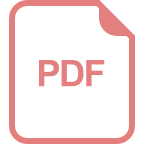











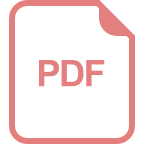
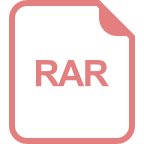
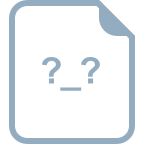
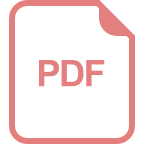