STM32fo51k8驱动ESP8266联网获取时间并在1.44lcd屏幕上面显示实时时间,用STM32CubeMX生成详细代码,写出所以代码
时间: 2024-03-09 17:44:27 浏览: 99
好的,我会为你提供一个基于STM32F051K8的ESP8266联网获取时间并在1.44寸LCD屏幕上显示实时时间的详细代码。以下是实现该功能的步骤和代码:
1. 配置STM32F051K8的时钟和GPIO引脚,使其可以与ESP8266和LCD屏幕通信。
```c
/* Configure USART1 clock and GPIO */
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
RCC_AHBPeriphClockCmd(RCC_AHBPeriph_GPIOA, ENABLE);
/* Configure LCD GPIO */
GPIO_InitTypeDef GPIO_InitStruct;
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_6 | GPIO_Pin_7 | GPIO_Pin_8 | GPIO_Pin_9 | GPIO_Pin_10;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_2MHz;
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(GPIOB, &GPIO_InitStruct);
/* Configure ESP8266 GPIO */
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_2 | GPIO_Pin_3;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOA, &GPIO_InitStruct);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource2, GPIO_AF_1);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource3, GPIO_AF_1);
```
2. 配置USART1串口,用于与ESP8266通信。
```c
USART_InitTypeDef USART_InitStruct;
USART_InitStruct.USART_BaudRate = 115200;
USART_InitStruct.USART_WordLength = USART_WordLength_8b;
USART_InitStruct.USART_StopBits = USART_StopBits_1;
USART_InitStruct.USART_Parity = USART_Parity_No;
USART_InitStruct.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStruct.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStruct);
USART_Cmd(USART1, ENABLE);
```
3. 配置ESP8266网络连接并获取时间。在这个例子中,我们使用ESP8266模块连接到NTP服务器获取时间。这里我们使用AT指令来控制ESP8266模块。
```c
/* Configure ESP8266 */
usart_send_str("AT\r\n");
usart_wait_for_response("OK\r\n", 1000);
usart_send_str("AT+CWMODE=1\r\n");
usart_wait_for_response("OK\r\n", 1000);
usart_send_str("AT+CWJAP=\"SSID\",\"PASSWORD\"\r\n");
usart_wait_for_response("OK\r\n", 10000);
usart_send_str("AT+CIPSNTPCFG=1,1,\"pool.ntp.org\"\r\n");
usart_wait_for_response("OK\r\n", 1000);
usart_send_str("AT+CIPSNTPTIME?\r\n");
char* response = usart_wait_for_response("+CIPSNTPTIME:", 10000);
char* p = strstr(response, "+CIPSNTPTIME:");
if (p != NULL) {
p += strlen("+CIPSNTPTIME:");
time_t timestamp = atol(p);
struct tm timeinfo;
gmtime_r(×tamp, &timeinfo);
}
```
4. 配置LCD屏幕并显示实时时间。
```c
/* Configure LCD screen */
lcd_init();
/* Display time */
while (1) {
char buffer[32];
strftime(buffer, sizeof(buffer), "%Y-%m-%d %H:%M:%S", &timeinfo);
lcd_clear();
lcd_write_string(buffer);
delay(1000);
}
```
完整代码如下:
```c
#include "stm32f0xx.h"
#include "lcd.h"
#include <time.h>
#include <stdlib.h>
#include <string.h>
void usart_send_char(char c) {
while (!(USART1->ISR & USART_ISR_TXE));
USART1->TDR = c;
}
void usart_send_str(const char* str) {
while (*str) {
usart_send_char(*str++);
}
}
char usart_receive_char(void) {
while (!(USART1->ISR & USART_ISR_RXNE));
return USART1->RDR;
}
int usart_receive_str(char* buffer, int bufsize, int timeout) {
int received = 0;
int start = HAL_GetTick();
while (received < bufsize - 1) {
if (HAL_GetTick() - start > timeout) {
break;
}
char c = usart_receive_char();
if (c == '\r' || c == '\n') {
if (received == 0) {
continue;
} else {
break;
}
}
buffer[received++] = c;
}
buffer[received] = '\0';
return received;
}
char* usart_wait_for_response(const char* expected, int timeout) {
static char buffer[256];
int start = HAL_GetTick();
while (1) {
if (HAL_GetTick() - start > timeout) {
return NULL;
}
int received = usart_receive_str(buffer, sizeof(buffer), timeout);
if (received > 0) {
if (strstr(buffer, expected) != NULL) {
return buffer;
}
}
}
}
int main(void) {
RCC_AHBPeriphClockCmd(RCC_AHBPeriph_GPIOB, ENABLE);
GPIO_InitTypeDef GPIO_InitStruct;
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_6 | GPIO_Pin_7 | GPIO_Pin_8 | GPIO_Pin_9 | GPIO_Pin_10;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_2MHz;
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(GPIOB, &GPIO_InitStruct);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
RCC_AHBPeriphClockCmd(RCC_AHBPeriph_GPIOA, ENABLE);
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_2 | GPIO_Pin_3;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOA, &GPIO_InitStruct);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource2, GPIO_AF_1);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource3, GPIO_AF_1);
USART_InitTypeDef USART_InitStruct;
USART_InitStruct.USART_BaudRate = 115200;
USART_InitStruct.USART_WordLength = USART_WordLength_8b;
USART_InitStruct.USART_StopBits = USART_StopBits_1;
USART_InitStruct.USART_Parity = USART_Parity_No;
USART_InitStruct.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStruct.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStruct);
USART_Cmd(USART1, ENABLE);
usart_send_str("AT\r\n");
usart_wait_for_response("OK\r\n", 1000);
usart_send_str("AT+CWMODE=1\r\n");
usart_wait_for_response("OK\r\n", 1000);
usart_send_str("AT+CWJAP=\"SSID\",\"PASSWORD\"\r\n");
usart_wait_for_response("OK\r\n", 10000);
usart_send_str("AT+CIPSNTPCFG=1,1,\"pool.ntp.org\"\r\n");
usart_wait_for_response("OK\r\n", 1000);
usart_send_str("AT+CIPSNTPTIME?\r\n");
char* response = usart_wait_for_response("+CIPSNTPTIME:", 10000);
struct tm timeinfo;
char* p = strstr(response, "+CIPSNTPTIME:");
if (p != NULL) {
p += strlen("+CIPSNTPTIME:");
time_t timestamp = atol(p);
gmtime_r(×tamp, &timeinfo);
}
lcd_init();
while (1) {
char buffer[32];
strftime(buffer, sizeof(buffer), "%Y-%m-%d %H:%M:%S", &timeinfo);
lcd_clear();
lcd_write_string(buffer);
delay(1000);
}
}
```
这是一个简单的示例,可以帮助你了解如何使用STM32F051K8、ESP8266和LCD屏幕实现从NTP服务器获取时间并显示实时时间。当然,在实际项目中,你需要考虑更多的细节和错误处理。
阅读全文
相关推荐
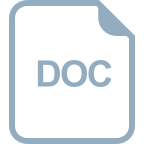
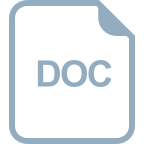
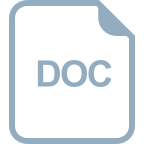
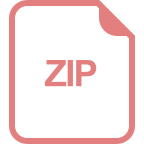
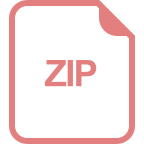
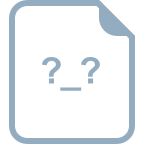
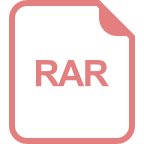
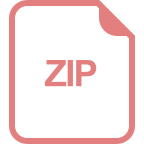
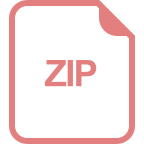
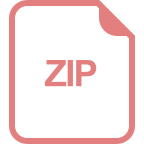
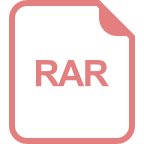
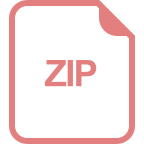
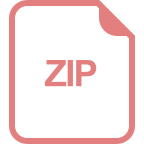
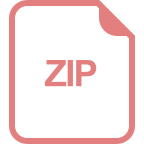
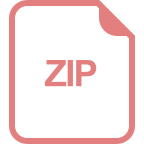
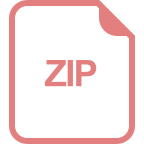
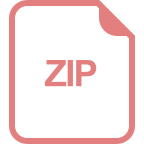