python对两组二维坐标进行仿射变换
时间: 2023-05-29 20:06:29 浏览: 226
可以使用OpenCV库对两组二维坐标进行仿射变换。下面是一个简单的示例代码:
```python
import numpy as np
import cv2
# 定义源坐标系和目标坐标系
src_pts = np.float32([[50, 50], [200, 50], [50, 200]])
dst_pts = np.float32([[10, 100], [200, 50], [100, 250]])
# 计算仿射变换矩阵
M = cv2.getAffineTransform(src_pts, dst_pts)
# 加载图像并应用仿射变换
img = cv2.imread('input.jpg')
rows, cols, _ = img.shape
img_affine = cv2.warpAffine(img, M, (cols, rows))
# 显示结果
cv2.imshow('Input', img)
cv2.imshow('Affine', img_affine)
cv2.waitKey(0)
```
在上面的示例代码中,我们首先定义了源坐标系和目标坐标系。然后使用`cv2.getAffineTransform()`函数计算仿射变换矩阵。接着加载图像,使用`cv2.warpAffine()`函数将图像应用仿射变换,并在窗口中显示结果。
相关问题
用python通过一致点来计算一个二维仿射变换矩阵
在计算二维仿射变换矩阵时,可以通过三个点来确定变换矩阵。如果这三个点分别为原图像上的 $(x_1, y_1)$,$(x_2, y_2)$,$(x_3, y_3)$ 和变换后的图像上的 $(u_1, v_1)$,$(u_2, v_2)$,$(u_3, v_3)$,则可以通过以下步骤来计算仿射变换矩阵:
1. 构造矩阵 $A$:
$$
A=\begin{bmatrix}
x_1 & y_1 & 1 & 0 & 0 & 0 \\
x_2 & y_2 & 1 & 0 & 0 & 0 \\
x_3 & y_3 & 1 & 0 & 0 & 0 \\
0 & 0 & 0 & x_1 & y_1 & 1 \\
0 & 0 & 0 & x_2 & y_2 & 1 \\
0 & 0 & 0 & x_3 & y_3 & 1 \\
\end{bmatrix}
$$
2. 构造矩阵 $B$:
$$
B=\begin{bmatrix}
u_1 \\
u_2 \\
u_3 \\
v_1 \\
v_2 \\
v_3 \\
\end{bmatrix}
$$
3. 解线性方程组 $Ax=B$,得到向量 $x=[a_{11}, a_{12}, a_{13}, a_{21}, a_{22}, a_{23}]$。
4. 将向量 $x$ 转换成仿射变换矩阵:
$$
M=\begin{bmatrix}
a_{11} & a_{12} & a_{13} \\
a_{21} & a_{22} & a_{23} \\
0 & 0 & 1 \\
\end{bmatrix}
$$
下面是使用 Python 实现上述算法的示例代码:
```python
import numpy as np
def find_affine_transform(src_points, dst_points):
# 构造矩阵 A 和 B
A = np.zeros((6, 6))
B = np.zeros((6, 1))
for i in range(3):
x, y = src_points[i]
u, v = dst_points[i]
A[i*2] = [x, y, 1, 0, 0, 0]
A[i*2+1] = [0, 0, 0, x, y, 1]
B[i*2] = u
B[i*2+1] = v
# 解线性方程组
x, _, _, _ = np.linalg.lstsq(A, B, rcond=None)
# 将向量 x 转换成仿射变换矩阵
M = np.array([[x[0], x[1], x[2]],
[x[3], x[4], x[5]],
[0, 0, 1]])
return M
```
其中,`src_points` 是原图像上的三个点的坐标,`dst_points` 是变换后的图像上的三个点的坐标,两个参数都是一个二维数组,每个元素是一个长度为 2 的一维数组,表示点的横坐标和纵坐标。函数返回的是一个 3x3 的 NumPy 数组,表示计算得到的仿射变换矩阵。
如果要对一个汉字进行仿射变换到两条曲线组成的区域内,已知该区域的四个端点,要求汉字要按照曲线的走向进行仿射变换,并将变换后的汉字进行显示,给出可运行的python代码
首先,你需要安装`Pillow`库(它是`PIL`的更现代版本),如果你还没有安装,可以使用`pip install pillow`命令进行安装。以下是一个简单的例子,展示了如何根据指定的四点边界对汉字进行仿射变换并显示出来。在这个示例中,我们将使用`PIL`的`ImageFont`、`ImageDraw`和`AffineTransform`模块。
```python
from PIL import Image, ImageFont, ImageDraw, ImageTransform
import numpy as np
def draw_char_within_curve(char, start_point, end_points):
# 创建字体和画布
font = ImageFont.truetype('simhei.ttf', size=30) # 替换为你的汉字字体文件
text_width, text_height = font.getsize(char)
# 创建初始的白色字符图像
transformed_char = Image.new("RGBA", (text_width, text_height), color=(255, 255, 255, 0))
draw = ImageDraw.Draw(transformed_char)
# 将字符转换为掩码,以便只填充黑色像素
mask = Image.new("L", (text_width, text_height), 0)
draw.text((0, 0), char, fill=255, font=font)
transformed_char.paste(mask, (0, 0), mask=mask)
# 创建仿射变换
affine = AffineTransform()
points = [start_point] + list(end_points)
for i in range(1, len(points)):
start = points[i - 1]
end = points[i]
x_diff = end[0] - start[0]
y_diff = end[1] - start[1]
if abs(x_diff) > abs(y_diff): # 按照x坐标方向调整变换
scale = abs(x_diff) / text_width
else:
scale = abs(y_diff) / text_height
rotation_angle = np.arctan2(y_diff, x_diff)
affine = AffineTransform(scale=scale, rotate=rotation_angle, translate=end)
# 应用仿射变换
transformed_char = transformed_char.transform((text_width, text_height), fillcolor='black', expand=True, offset=(0, 0), affinity=affine)
return transformed_char
# 使用示例:假设你有四个端点(这里以二维坐标表示)
start_point = (100, 100) # 曲线起点
end_points = [(150, 120), (200, 150), (180, 180)] # 曲线终点
char_to_transform = '你好'
transformed_char = draw_char_within_curve(char_to_transform, start_point, end_points)
# 展示变换后的字符
transformed_char.show()
阅读全文
相关推荐
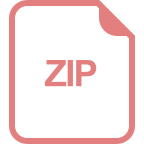
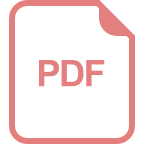
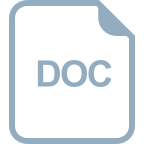
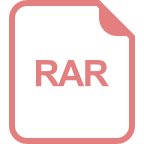
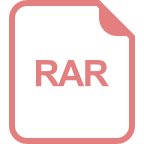
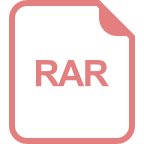
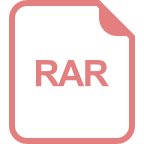
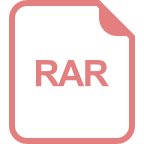
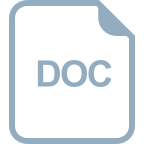
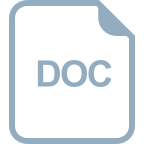
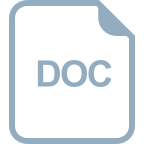
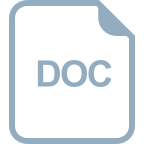
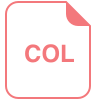


