TypeError: stack(): argument 'tensors' (position 1) must be tuple of Tensors, not Tensor
时间: 2024-04-29 13:23:23 浏览: 336
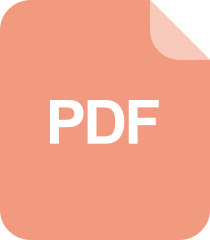
解决Python中报错TypeError: must be str, not bytes问题

This error occurs when the `stack()` function in PyTorch is called with a single tensor instead of a tuple of tensors.
To resolve this error, you need to pass a tuple of tensors to the `stack()` function instead of a single tensor. For example:
```
import torch
# Create two tensors
tensor1 = torch.tensor([1, 2, 3])
tensor2 = torch.tensor([4, 5, 6])
# Use stack() function to stack the tensors
stacked_tensors = torch.stack((tensor1, tensor2))
print(stacked_tensors)
```
This will output:
```
tensor([[1, 2, 3],
[4, 5, 6]])
```
Notice that we passed a tuple of tensors `(tensor1, tensor2)` to the `stack()` function.
阅读全文
相关推荐
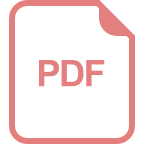















