修改Fraction类的构造方法,使其检查并确保分子和分母为整数。如果任一不是整数,就抛出异常,同时实现下列简单的算术运算:__sub__、__mul__、__truediv__,以及下列关系运算:__gt__、__ge__、__lt__、__le__和__ne__
时间: 2023-10-24 15:04:55 浏览: 133
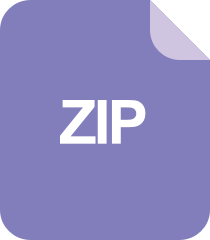
设计一个表示分数的类Fraction。这个类用两个int类型的变量分别表示分子和分母。

好的,你可以参考下面的代码来修改Fraction类的构造方法并实现所需的算术和关系运算:
```python
class Fraction:
def __init__(self, numerator, denominator):
if not isinstance(numerator, int) or not isinstance(denominator, int):
raise ValueError("分子和分母必须为整数")
if denominator == 0:
raise ValueError("分母不能为零")
self.numerator = numerator
self.denominator = denominator
def simplify(self):
gcd = self.gcd(self.numerator, self.denominator)
self.numerator //= gcd
self.denominator //= gcd
@staticmethod
def gcd(a, b):
while b != 0:
a, b = b, a % b
return a
def __str__(self):
return f"{self.numerator}/{self.denominator}"
def __sub__(self, other):
new_numerator = self.numerator * other.denominator - other.numerator * self.denominator
new_denominator = self.denominator * other.denominator
result = Fraction(new_numerator, new_denominator)
result.simplify()
return result
def __mul__(self, other):
new_numerator = self.numerator * other.numerator
new_denominator = self.denominator * other.denominator
result = Fraction(new_numerator, new_denominator)
result.simplify()
return result
def __truediv__(self, other):
new_numerator = self.numerator * other.denominator
new_denominator = self.denominator * other.numerator
result = Fraction(new_numerator, new_denominator)
result.simplify()
return result
def __gt__(self, other):
return self.numerator * other.denominator > other.numerator * self.denominator
def __ge__(self, other):
return self.numerator * other.denominator >= other.numerator * self.denominator
def __lt__(self, other):
return self.numerator * other.denominator < other.numerator * self.denominator
def __le__(self, other):
return self.numerator * other.denominator <= other.numerator * self.denominator
def __ne__(self, other):
return self.numerator * other.denominator != other.numerator * self.denominator
# 测试代码
f1 = Fraction(1, 2)
f2 = Fraction(3, 4)
print(f1 - f2)
print(f1 * f2)
print(f1 / f2)
print(f1 > f2)
print(f1 >= f2)
print(f1 < f2)
print(f1 <= f2)
print(f1 != f2)
```
这个代码修改了构造方法,确保分子和分母为整数,如果不是整数则抛出异常。还实现了`__sub__`、`__mul__`、`__truediv__`等算术运算符重载方法,以及`__gt__`、`__ge__`、`__lt__`、`__le__`和`__ne__`等关系运算符重载方法。
阅读全文
相关推荐
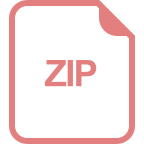















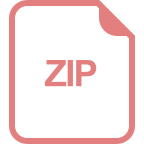