编写一个c语言程序,要求用户输入一段英语句子(例如Mechanical Ancestor is a team.),将其存储在一个字符数组中。(1).打印出这个数组总共有多少个字母 a(不分大小写),并输出这些字符在数组中的位置和地址。 (2).找出这个数组中最长的单词并打印。
时间: 2024-10-21 08:13:09 浏览: 21
首先,让我们创建一个C语言程序来完成这个任务。我们将使用`scanf`函数获取用户输入,然后遍历数组计算a字母的数量、它们的位置和地址,以及查找最长的单词。
```c
#include <stdio.h>
#include <ctype.h>
#define MAX_WORDS 10 // 预设最多单词数
#define MAX_LEN 50 // 字符串最大长度
// 定义结构体来存储单词信息
typedef struct {
char* word;
int start;
int length;
} Word;
int main() {
char sentence[MAX_LEN];
int letter_count = 0; // 记录'a'字母的数量
char last_space = '\0'; // 上一个空格的位置
Word words[MAX_WORDS]; // 存储单词的结构体数组
int num_words = 0; // 当前单词计数
printf("请输入一段英文句子: ");
fgets(sentence, sizeof(sentence), stdin); // 读取用户的输入
sentence[strcspn(sentence, "\n")] = '\0'; // 删除行尾的换行符
for (int i = 0; i < strlen(sentence); ++i) {
if (isalpha(sentence[i])) { // 检查是否是字母
if (tolower(sentence[i]) == 'a') { // 不区分大小写
letter_count++;
// 如果遇到空格,保存当前单词的信息
if (sentence[i] != ' ') {
words[num_words].word = &sentence[i - 1]; // 起始位置减一,因为索引从0开始
words[num_words].start = i - last_space;
}
}
if (sentence[i] == ' ') {
if (num_words > 0) {
words[num_words - 1].length = i - last_space; // 更新单词长度
num_words++; // 新增一个单词
}
last_space = i + 1;
}
}
}
words[num_words - 1].length = strlen(sentence) - last_space; // 最后一个单词的长度
printf("\n统计结果:\n");
printf("总字母a的数量: %d\n", letter_count);
for (int j = 0; j < num_words; j++) {
printf("单词 '%s', 开始位置: %d, 地址: %p\n", words[j].word, words[j].start, words[j].word);
}
// 找到最长的单词
int max_length_word_index = 0;
for (int k = 0; k < num_words; k++) {
if (words[k].length > words[max_length_word_index].length) {
max_length_word_index = k;
}
}
printf("最长单词: '%s'\n", words[max_length_word_index].word);
return 0;
}
```
运行此程序后,按照提示输入一个英文句子,它将打印字母"a"的数量及其位置,每个单词的位置和地址,并找到最长的单词。
阅读全文
相关推荐
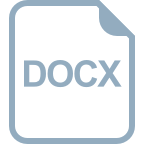
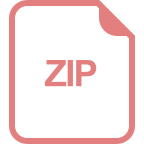
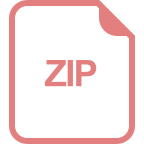
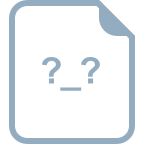
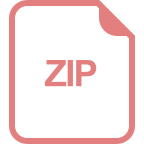
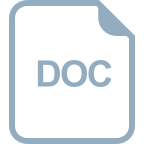
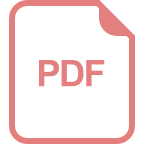











