写出c++链栈的基本操作和实现的完整代码
时间: 2024-10-18 18:16:04 浏览: 50
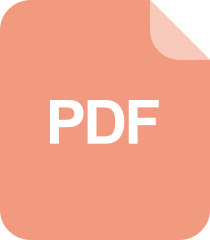
C++实现链栈的基本操作

链栈(Linked Stack)是一种基于链表的数据结构实现的栈,它使用链表头部作为栈顶元素。在C++中,我们可以创建一个Node类表示链表节点,然后定义Stack类来包含插入(push)、删除(pop)、查看栈顶元素(top)等基本操作。
以下是C++链栈的简单实现:
```cpp
#include <iostream>
// 链表节点类
class Node {
public:
int data;
Node* next;
Node(int val) : data(val), next(nullptr) {}
};
// 链栈类
class Stack {
private:
Node* top; // 栈顶指针
public:
// 初始化
Stack() : top(nullptr) {}
// 判断是否为空
bool isEmpty() const { return top == nullptr; }
// 入栈(push)
void push(int value) {
Node* newNode = new Node(value);
newNode->next = top;
top = newNode;
}
// 出栈(pop)
int pop() {
if (isEmpty()) {
throw std::runtime_error("Stack is empty");
}
int poppedValue = top->data;
Node* temp = top;
top = top->next;
delete temp;
return poppedValue;
}
// 查看栈顶元素(只读)
int peek() const {
if (isEmpty()) {
throw std::runtime_error("Stack is empty");
}
return top->data;
}
// 清空栈
void clear() {
while (!isEmpty()) {
pop();
}
}
};
int main() {
Stack s;
s.push(10);
s.push(20);
std::cout << "Top element: " << s.peek() << std::endl;
std::cout << "Popped element: " << s.pop() << std::endl;
阅读全文
相关推荐
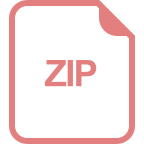
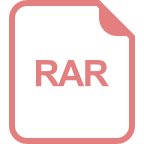


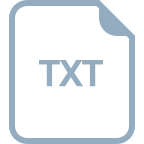
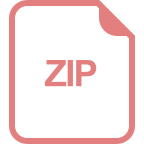
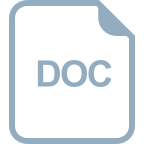
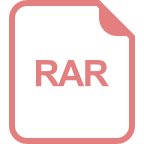





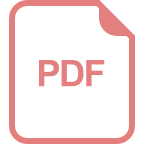
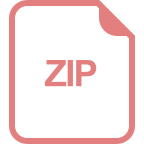
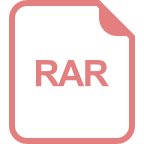
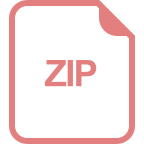
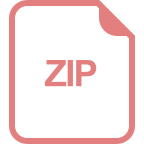