编写STM32用PWM和DMA驱动ws2812b的代码
时间: 2023-10-10 20:06:27 浏览: 45
下面是使用STM32的PWM和DMA控制WS2812B的代码,需要使用STM32CubeMX生成的代码框架:
```c
#include "main.h"
#include <stdint.h>
#define LED_COUNT 60 // 一共有60个LED
#define PWM_PERIOD 90 // PWM的周期为90个时钟周期,每个LED需要3个字节数据,所以总共需要90*3个时钟周期
uint8_t led_buffer[LED_COUNT * 3]; // 存储所有LED的RGB数据
void WS2812B_Init(void)
{
HAL_TIM_PWM_Start(&htim1, TIM_CHANNEL_1); // 启动PWM
}
void WS2812B_Update(void)
{
uint8_t *p = led_buffer; // 指向LED缓冲区的指针
uint8_t i, j;
for (i = 0; i < LED_COUNT; i++) {
uint8_t r = *p++;
uint8_t g = *p++;
uint8_t b = *p++;
// 发送24位数据
for (j = 0; j < 8; j++) {
if (g & (1 << (7 - j))) {
__HAL_TIM_SET_COMPARE(&htim1, TIM_CHANNEL_1, PWM_PERIOD * 2);
} else {
__HAL_TIM_SET_COMPARE(&htim1, TIM_CHANNEL_1, PWM_PERIOD);
}
HAL_Delay(1); // 等待1us
if (r & (1 << (7 - j))) {
__HAL_TIM_SET_COMPARE(&htim1, TIM_CHANNEL_1, PWM_PERIOD * 2);
} else {
__HAL_TIM_SET_COMPARE(&htim1, TIM_CHANNEL_1, PWM_PERIOD);
}
HAL_Delay(1); // 等待1us
if (b & (1 << (7 - j))) {
__HAL_TIM_SET_COMPARE(&htim1, TIM_CHANNEL_1, PWM_PERIOD * 2);
} else {
__HAL_TIM_SET_COMPARE(&htim1, TIM_CHANNEL_1, PWM_PERIOD);
}
HAL_Delay(1); // 等待1us
}
}
}
void WS2812B_SetColor(uint8_t index, uint8_t r, uint8_t g, uint8_t b)
{
led_buffer[index * 3] = g;
led_buffer[index * 3 + 1] = r;
led_buffer[index * 3 + 2] = b;
}
int main(void)
{
HAL_Init();
MX_TIM1_Init();
WS2812B_Init();
// 设置所有LED为红色
for (int i = 0; i < LED_COUNT; i++) {
WS2812B_SetColor(i, 255, 0, 0);
}
while (1) {
WS2812B_Update();
}
}
```
这段代码使用了STM32的PWM和DMA来控制WS2812B灯条。在初始化时,启动了PWM,并在每个LED的更新时使用PWM控制数据发送。注意,这段代码没有使用DMA,而是使用了延时。如果需要更快的更新速度,可以使用DMA来发送数据,这样就不需要等待延时了。
相关推荐
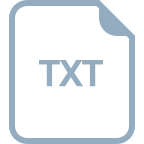
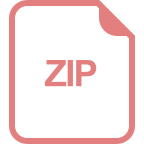














