使用c语言完成下列要求:1.写一函数实现根据给定的一组整数创建BST树; 2.写一函数实现在BST树上查找指定的数据; 在主函数中调用创建BST树的函数,并针对某些数据调用查找函数进行查找,并把查找结果向屏幕输出。
时间: 2024-03-15 13:44:01 浏览: 25
好的,以下是使用C语言实现的代码。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树节点结构体
struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
};
// 创建新节点
struct TreeNode* new_node(int val) {
struct TreeNode* node = (struct TreeNode*) malloc(sizeof(struct TreeNode));
node->val = val;
node->left = NULL;
node->right = NULL;
return node;
}
// 插入节点
struct TreeNode* insert_node(struct TreeNode* root, int val) {
if (root == NULL) {
return new_node(val);
}
if (val < root->val) {
root->left = insert_node(root->left, val);
}
else {
root->right = insert_node(root->right, val);
}
return root;
}
// 创建BST树
struct TreeNode* create_BST(int* nums, int numsSize) {
struct TreeNode* root = NULL;
for (int i = 0; i < numsSize; i++) {
root = insert_node(root, nums[i]);
}
return root;
}
// 查找节点
struct TreeNode* search_node(struct TreeNode* root, int val) {
if (root == NULL || root->val == val) {
return root;
}
if (val < root->val) {
return search_node(root->left, val);
}
else {
return search_node(root->right, val);
}
}
int main() {
int nums[] = {5, 3, 8, 4, 1, 9, 2, 7, 6};
int numsSize = sizeof(nums) / sizeof(nums[0]);
struct TreeNode* root = create_BST(nums, numsSize);
int target = 4;
struct TreeNode* node = search_node(root, target);
if (node != NULL) {
printf("Found %d in BST.\n", target);
}
else {
printf("Did not find %d in BST.\n", target);
}
return 0;
}
```
以上代码实现了创建一组整数的BST树,并在BST树上查找指定的数据。你可以根据自己的需要修改数据,比如要查找的数据和要创建的整数组等。运行代码,你将得到查找结果。
相关推荐
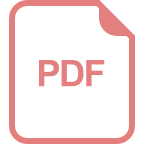














