我需要俄罗斯方块c++代码
时间: 2024-11-15 21:27:41 浏览: 0
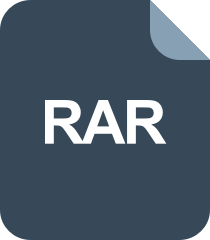
俄罗斯方块C++源代码

创建一个完整的俄罗斯方块游戏是一个相当复杂的项目,涉及到图形界面、游戏逻辑、碰撞检测等多个方面。由于这是一个大型的主题,这里我会给你提供一个简化的框架代码,你可以从这个开始扩展。以下是一个基本的游戏循环和方块类的概要:
```cpp
#include <iostream>
#include <vector>
// 定义一个简单的方块类
class Block {
public:
enum Shape { I, J, L, O, S, T, Z };
Block(int x, int y, Shape shape) : position{x, y}, shape(shape) {}
// 获取方块的位置和形状
int getX() const { return position.x; }
int getY() const { return position.y; }
Shape getShape() const { return shape; }
private:
std::pair<int, int> position;
Shape shape;
};
// 简化版的游戏循环(不包含图形显示)
void gameLoop(std::vector<Block>& blocks, int boardWidth, int boardHeight) {
while (true) {
// 更新玩家操作
// ...
// 检查是否满行
bool fullRow = checkFullRows(blocks, boardHeight);
if (fullRow) {
removeFullRows(blocks);
}
// 移动或旋转方块
// ...
}
}
// 检查一行是否满了
bool checkFullRows(const std::vector<Block>& blocks, int boardHeight) {
for (int i = 0; i < boardHeight; ++i) {
if (!anyBlockInRow(blocks, i)) {
return false;
}
}
return true;
}
// 假设anyBlockInRow是一个检查某行是否有方块的方法
bool anyBlockInRow(const std::vector<Block>& blocks, int row) {
for (const auto& block : blocks) {
if (block.getY() == row) {
return true;
}
}
return false;
}
// 其他辅助功能如移除满行等...
```
这只是一个非常基础的框架,真正的俄罗斯方块游戏还需要处理游戏状态的保存/加载、键盘输入、动画效果、得分计算以及图形渲染等。如果你是初学者,可以从这里开始构建并逐步添加复杂性。
阅读全文
相关推荐
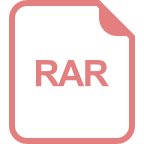
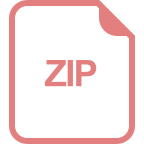
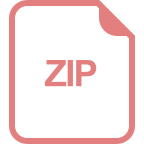
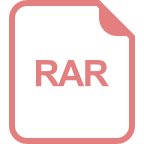
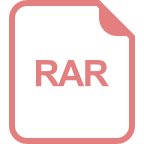

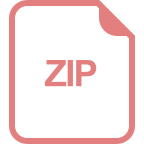