springboot下 java excel数据导入开发
时间: 2024-10-23 13:02:54 浏览: 30
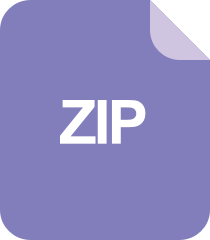
使用Springboot实现excel的导入导出
Spring Boot 下使用 Java Excel 进行数据导入的开发通常涉及以下几个步骤:
1. 添加依赖:首先,需要在项目的 Maven 或者 Gradle 依赖管理中添加 Apache POI 或者 FasterXML 的 Jackson-Excel 工具包,它们分别用于读取和操作 Excel 文件。
Maven 示例:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.opencsv</groupId>
<artifactId>opencsv</artifactId>
</dependency>
<!-- 或者 Jackson-Excel -->
<dependency>
<groupId>com.fasterxml.jackson.dataformat</groupId>
<artifactId>jackson-dataformat-excel</artifactId>
</dependency>
```
2. 创建 Excel 导入服务:创建一个服务类,例如 `ExcelImportService`,使用 Spring 的 @Autowired 注解注入一个读取 Excel 的工具类,如 `FileUtils` 来处理文件上传和读取。
3. 定义映射:定义一个 Java 对象来映射 Excel 表格的数据,比如 `UserExcelEntity`,它包含属性与 Excel 行对应的字段。
4. 读取 Excel:使用工具库提供的 API,如 `importUsersFromExcel()` 方法,解析 Excel 文件并将数据转换为你定义的对象列表。
```java
List<UserExcelEntity> entities = new ArrayList<>();
try {
Workbook workbook = readExcelFile(fileName);
for (Sheet sheet : workbook.getSheets()) {
Iterator<Row> iterator = sheet.iterator();
while (iterator.hasNext()) {
Row row = iterator.next();
UserExcelEntity entity = mapRowToEntity(row);
entities.add(entity);
}
}
} catch (IOException e) {
log.error("Error reading Excel file", e);
}
```
5. 转换数据:`mapRowToEntity(Row row)` 方法将每一行 Excel 数据转换为 `UserExcelEntity` 对象。
6. 存储数据:如果需要,将 `UserExcelEntity` 列表保存到数据库或者其他存储系统,或者直接进行业务处理。
阅读全文
相关推荐
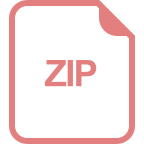
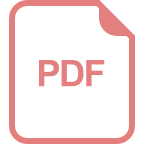
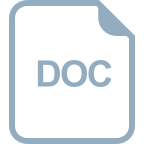
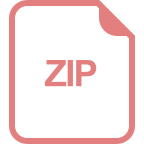
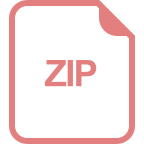











