windows opencv 推流 c++
时间: 2023-06-13 08:05:44 浏览: 75
要在 Windows 上使用 OpenCV 推流,你需要使用相应的库和工具。以下是一个基本的示例代码,可以将 OpenCV 的视频流推送到 RTMP 服务器。
首先,你需要使用 CMake 来构建和安装 OpenCV 库。请注意,你需要启用 FFmpeg 和 RTMP 支持。然后,你需要安装 RTMP 服务器,例如 nginx 或 Wowza。
接下来,我们来看看如何使用 OpenCV 推送 RTMP 流。你需要使用 FFmpeg 和 libavcodec 库来将视频压缩并推送到服务器。在 Windows 上,你可以使用 vcpkg 作为包管理器,安装这些库。
接下来是示例代码:
```c++
#include <opencv2/opencv.hpp>
#include <opencv2/highgui/highgui.hpp>
#include <opencv2/imgproc/imgproc.hpp>
#include <opencv2/videoio.hpp>
extern "C"
{
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libavutil/opt.h>
#include <libswscale/swscale.h>
}
using namespace cv;
int main()
{
// Open video capture device
VideoCapture cap(0);
if (!cap.isOpened())
{
std::cerr << "Error opening video capture device" << std::endl;
return -1;
}
// Set up output stream
const char* rtmpUrl = "rtmp://localhost/live/stream";
av_register_all();
avcodec_register_all();
AVFormatContext* pFormatCtx = avformat_alloc_context();
AVOutputFormat* fmt = av_guess_format("flv", rtmpUrl, NULL);
pFormatCtx->oformat = fmt;
AVCodec* codec = avcodec_find_encoder(AV_CODEC_ID_H264);
AVStream* video_st = avformat_new_stream(pFormatCtx, codec);
video_st->time_base.num = 1;
video_st->time_base.den = 25;
AVCodecContext* codecCtx = avcodec_alloc_context3(codec);
codecCtx->codec_id = codec->id;
codecCtx->codec_type = AVMEDIA_TYPE_VIDEO;
codecCtx->pix_fmt = AV_PIX_FMT_YUV420P;
codecCtx->width = 640;
codecCtx->height = 480;
codecCtx->bit_rate = 400000;
codecCtx->time_base.num = 1;
codecCtx->time_base.den = 25;
codecCtx->gop_size = 10;
codecCtx->max_b_frames = 1;
avcodec_open2(codecCtx, codec, NULL);
avcodec_parameters_from_context(video_st->codecpar, codecCtx);
avio_open(&pFormatCtx->pb, rtmpUrl, AVIO_FLAG_WRITE);
// Start capturing and streaming
Mat frame;
AVFrame* avFrame = av_frame_alloc();
avFrame->format = AV_PIX_FMT_YUV420P;
avFrame->width = codecCtx->width;
avFrame->height = codecCtx->height;
av_frame_get_buffer(avFrame, 32);
SwsContext* swsCtx = sws_getContext(codecCtx->width, codecCtx->height, AV_PIX_FMT_BGR24,
codecCtx->width, codecCtx->height, AV_PIX_FMT_YUV420P,
SWS_BILINEAR, NULL, NULL, NULL);
while (true)
{
cap.read(frame);
if (frame.empty())
break;
// Convert frame to YUV
Mat yuv;
cvtColor(frame, yuv, COLOR_BGR2YUV_I420);
// Scale frame to output size
AVPicture picture;
picture.data[0] = avFrame->data[0];
picture.data[1] = avFrame->data[1];
picture.data[2] = avFrame->data[2];
picture.linesize[0] = avFrame->linesize[0];
picture.linesize[1] = avFrame->linesize[1];
picture.linesize[2] = avFrame->linesize[2];
sws_scale(swsCtx, yuv.data, yuv.step, 0, codecCtx->height, picture.data, picture.linesize);
// Encode and write frame
AVPacket pkt;
av_init_packet(&pkt);
pkt.data = NULL;
pkt.size = 0;
avcodec_send_frame(codecCtx, avFrame);
while (avcodec_receive_packet(codecCtx, &pkt) == 0)
{
pkt.stream_index = video_st->index;
av_packet_rescale_ts(&pkt, codecCtx->time_base, video_st->time_base);
av_interleaved_write_frame(pFormatCtx, &pkt);
}
}
// Clean up
av_write_trailer(pFormatCtx);
avcodec_close(codecCtx);
avcodec_free_context(&codecCtx);
av_frame_free(&avFrame);
sws_freeContext(swsCtx);
avio_close(pFormatCtx->pb);
avformat_free_context(pFormatCtx);
return 0;
}
```
这个示例假设你的摄像头输出格式是 BGR24,视频大小为 640x480,帧率为 25fps,码率为 400 kbps。你需要根据自己的设置进行调整。要编译这个示例,你需要将 OpenCV 和 FFmpeg 的库链接到你的项目中。
我希望这可以帮助你入门 OpenCV 的推流功能。
相关推荐
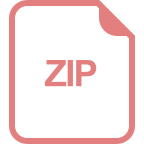
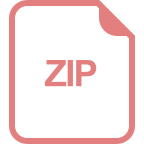














