帮我写一段多元logit模型采用极大似然估计方法估计参数用python写
时间: 2023-05-26 12:01:24 浏览: 72
Sure! Here is an example of how to estimate the parameters of a multinomial logit model using maximum likelihood estimation in python:
```
import numpy as np
from scipy.optimize import minimize
# Define the log-likelihood function for the multinomial logit model
def log_likelihood(params, X, y):
beta = params[:-1]
gamma = params[-1]
n_classes = np.unique(y).shape[0]
utility = np.dot(X, beta) + gamma
log_likelihood = 0
for i in range(X.shape[0]):
den = 0
for j in range(n_classes):
den += np.exp(utility[i, j])
log_likelihood += np.log(np.exp(utility[i, y[i]]) / den)
return -log_likelihood
# Set up the input data
X = np.random.randn(100, 3)
y = np.random.randint(0, 3, 100)
# Set up the initial parameter values and bounds
beta_init = np.zeros((X.shape[1], 1))
gamma_init = np.zeros((1, 1))
params_init = np.vstack((beta_init, gamma_init))
bounds = [(None, None)] * X.shape[1] + [(None, None)]
bounds[-1] = (None, None)
# Use the Scipy minimize function to estimate the parameters
result = minimize(log_likelihood, params_init, args=(X, y), bounds=bounds)
params_ml = result.x
# Print the estimated parameters
beta_ml = params_ml[:-1]
gamma_ml = params_ml[-1]
print("Estimated beta:", beta_ml)
print("Estimated gamma:", gamma_ml)
```
In this example, we start by defining the log-likelihood function for the multinomial logit model. This function takes as input the model parameters, `params`, the input data `X` and the output labels `y`. The function calculates the utility for each class for each observation, and then calculates the log-likelihood of the model given the observed data.
We then set up the input data, `X` and `y`, and the initial parameter values and bounds. We use the `minimize` function from the `scipy.optimize` module to find the maximum likelihood estimates of the parameters. The `minimize` function takes as input the log-likelihood function, the initial parameter values, and any additional arguments needed by the log-likelihood function.
Finally, we print out the estimated parameters, `beta_ml` and `gamma_ml`. These parameters represent the coefficients for the input variables and the intercept term, respectively.
相关推荐
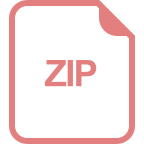
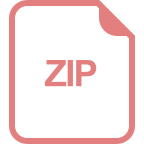
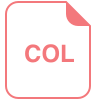













