单链表平方和的递归算法
时间: 2024-11-12 11:10:11 浏览: 14
单链表平方和的递归算法通常是用来解决这样一个问题:给定一个非空的整数链表,它的每个节点包含一个整数值,你需要计算所有节点值的平方之和。递归的一种解决方案是将这个问题分解成两个子问题:首先递归地处理列表的前半部分,然后递归地处理剩余的后半部分,最后将这两个结果相加。
以下是基本的递归思路:
1. **基本情况**: 如果链表只有一个元素,那么返回该元素的平方。
2. **递归情况**: 对于长度大于1的链表,将其拆分为两部分,对前半部分进行递归求和(`sumOfSquares(head)`),然后对后半部分也进行递归求和(`sumOfSquares(cycle.next)`),最后将两者的结果相加:`head.val * head.val + sumOfSquares(head.next)`。
这是一个典型的分治策略,在Python中可以这样实现:
```python
def squareSum(head):
if not head or not head.next:
return head.val**2 if head else 0 # 基本情况
slow = fast = head
cycle_start = None
while fast and fast.next:
slow = slow.next
fast = fast.next.next
if slow == fast: # 检查是否有环
cycle_start = slow
break
if cycle_start:
# 计算环内的节点总和
loop_sum = 0
current = cycle_start
while current != slow:
loop_sum += current.val
current = current.next
non_cycle_head = slow.next # 跳过循环部分
else:
non_cycle_head = head
# 递归处理前半部分和后半部分
half_length = len(non_cycle_head) // 2
first_half = squareSum(head) if half_length > 0 else 0
second_half = squareSum(cycle_start.next) if cycle_start else 0
# 返回总和
return head.val * head.val + first_half + second_half + (loop_sum * loop_sum)
```
阅读全文
相关推荐





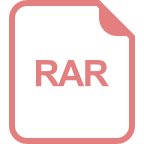

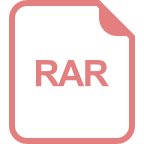






