windows使用c++ + ffmpeg打开笔记本电脑的摄像头
时间: 2023-08-30 10:02:01 浏览: 266
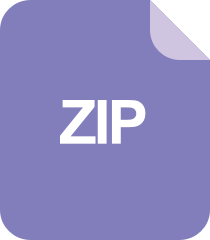
c++调用笔记本电脑自带摄像头或外接摄像头,后续会实现截图保存功能,平台为VC6.0.zip
要使用C语言和FFmpeg打开笔记本电脑的摄像头,首先需要在Windows系统下安装好FFmpeg库。安装完成后,可以使用以下代码来实现:
1. 首先,包含FFmpeg的头文件和其他必要的库文件。
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdint.h>
#include <string.h>
#include <windows.h>
#include <libavcodec/avcodec.h>
#include <libavdevice/avdevice.h>
#include <libavformat/avformat.h>
#include <libswscale/swscale.h>
```
2. 初始化FFmpeg并打开摄像头。
```c
int main() {
// 初始化FFmpeg
av_register_all();
avformat_network_init();
avdevice_register_all();
AVFormatContext* formatContext = NULL;
// 打开摄像头
AVInputFormat* inputFormat = av_find_input_format("dshow");
avformat_open_input(&formatContext, "video=Integrated Webcam", inputFormat, NULL);
avformat_find_stream_info(formatContext, NULL);
// 查找并打开视频流
int videoStream = -1;
for (int i = 0; i < formatContext->nb_streams; i++) {
if (formatContext->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
videoStream = i;
break;
}
}
if (videoStream == -1) {
printf("无法打开视频流。\n");
return -1;
}
// 读取视频帧
AVPacket packet;
av_init_packet(&packet);
AVCodecContext* codecContext = formatContext->streams[videoStream]->codec;
AVCodec* codec = avcodec_find_decoder(codecContext->codec_id);
avcodec_open2(codecContext, codec, NULL);
AVFrame* frame = av_frame_alloc();
AVFrame* frameRGB = av_frame_alloc();
int numBytes = avpicture_get_size(AV_PIX_FMT_RGB24, codecContext->width, codecContext->height);
uint8_t* buffer = (uint8_t*)av_malloc(numBytes * sizeof(uint8_t));
avpicture_fill((AVPicture*)frameRGB, buffer, AV_PIX_FMT_RGB24, codecContext->width, codecContext->height);
struct SwsContext* swsContext = sws_getContext(
codecContext->width,
codecContext->height,
codecContext->pix_fmt,
codecContext->width,
codecContext->height,
AV_PIX_FMT_RGB24,
SWS_BILINEAR,
NULL,
NULL,
NULL
);
while (av_read_frame(formatContext, &packet) >= 0) {
if (packet.stream_index == videoStream) {
avcodec_decode_video2(codecContext, frame, &frameFinished, &packet);
if (frameFinished) {
sws_scale(
swsContext,
frame->data,
frame->linesize,
0,
codecContext->height,
frameRGB->data,
frameRGB->linesize
);
// 在这里可以对图像帧进行处理
}
}
av_packet_unref(&packet);
}
// 清理资源
av_frame_free(&frame);
av_frame_free(&frameRGB);
avcodec_close(codecContext);
avformat_close_input(&formatContext);
avformat_network_deinit();
return 0;
}
```
这个代码片段会打开笔记本电脑的摄像头,读取摄像头返回的图像帧,并将其存储在RGB格式的帧中。你可以根据需要,在代码中加入对图像帧的处理逻辑。最后,记得清理资源并关闭摄像头。
注意:这只是一个简单的示例,实际应用中可能需要处理更多的异常情况和错误处理。另外,由于某些Windows系统使用的摄像头驱动不兼容FFmpeg,可能需要额外的配置和处理才能正常工作。
阅读全文
相关推荐
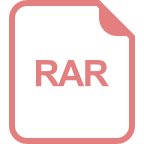
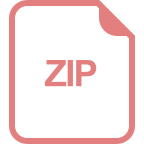
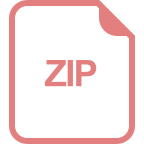
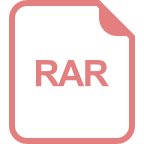
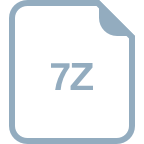
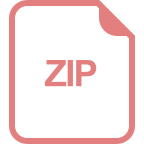
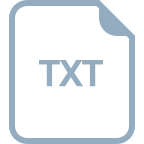
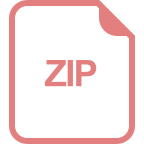
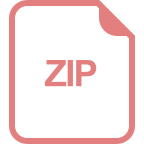
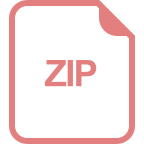
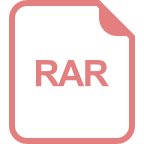
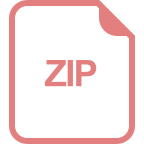
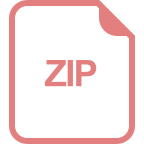
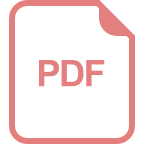
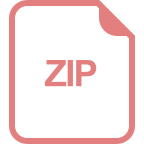