import json import os with open('file_1.json', 'r',encoding='utf-8') as f: data = json.load(f) # print(data) path=r"C:\Users\admin\Desktop\weapon_all_name" image_folder = path image_files = [f for f in os.listdir(image_folder) if os.path.isfile(os.path.join(image_folder, f))] matched_files = [] for file in image_files: filename = os.path.splitext(file)[1] if filename in data: matched_files.append(file) for file in matched_files: print(file)
时间: 2023-12-13 10:03:01 浏览: 46
您的代码段看起来很好!这段代码首先使用`json`模块加载名为`file_1.json`的JSON文件中的数据,并将其存储在变量`data`中。
然后,您定义了文件夹路径`path`,并将其赋值给变量`image_folder`。接下来,使用列表推导式和`os.listdir()`函数获取指定文件夹中的所有文件的名称,并将它们存储在`image_files`列表中。
接下来,代码使用循环遍历`image_files`列表中的每个文件,使用`os.path.splitext()`函数获取文件的扩展名,并将其存储在变量`filename`中。然后,检查`filename`是否存在于`data`字典中,如果存在,则将该文件名添加到`matched_files`列表中。
最后,通过循环遍历`matched_files`列表,并打印每个文件名。
如果您有其他问题,请随时提问!
相关问题
with open('simulation/case' + str(i) + "/controlData.json", "r", encoding='utf-8') as jsonFile:
您想了解如何使用Python中的open函数来打开JSON文件并读取其中的内容。在这个例子中,文件路径是通过字符串拼接来创建的。变量i是一个整数,通过str()函数将其转换为字符串,然后与其他字符串拼接。文件以只读模式打开,并指定编码为utf-8。
接下来,您可以使用json模块中的load方法来加载JSON文件的内容。您可以将打开的文件对象作为参数传递给load方法,它将返回一个包含JSON数据的Python对象。以下是一个示例代码:
```python
import json
file_path = 'simulation/case' + str(i) + "/controlData.json"
with open(file_path, "r", encoding='utf-8') as json_file:
data = json.load(json_file)
# 现在您可以对data进行操作,它将包含JSON文件的内容
```
请注意,这里的data变量将包含JSON文件的内容,您可以根据需要对其进行操作。
108至116行代码为:Data_Path = r'G:\项目\RCM\RCM自\datafile\工单.json' Data_Pathout = r'G:\项目\RCM\RCM自\datafile\wangdatong.json' # ----------读取数据 with open(Data_Path, 'r', encoding='utf8') as load_f: Data_in = json.load(load_f) Data_in = json.dumps(Data_in, ensure_ascii=False) # ----------故障模式概率等级\故障影响等级\CA FMECA_out = FMECA(Data_in)
根据你提供的代码,我注意到在读取`Data_Path`指向的JSON文件后,你将其转换为字符串类型。然后你将这个字符串类型的数据传递给`FMECA`函数进行处理。
问题可能出现在这个转换过程中。将JSON字符串转换为字典之后,你应该直接使用字典类型的`Data_in`,而不是再次将其转换为字符串。
请尝试使用下面的修正后的代码:
```python
import json
Data_Path = r'G:\项目\RCM\RCM自\datafile\工单.json'
Data_Pathout = r'G:\项目\RCM\RCM自\datafile\wangdatong.json'
# 读取数据
with open(Data_Path, 'r', encoding='utf8') as load_f:
Data_in = json.load(load_f)
# 故障模式概率等级\故障影响等级\CA
FMECA_out = FMECA(Data_in)
```
这样,`Data_in`将是一个字典类型的变量,你可以将其直接传递给`FMECA`函数。
如果问题仍然存在,请提供更多的代码和错误信息,以便我能够更好地帮助你解决问题。
相关推荐
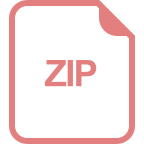
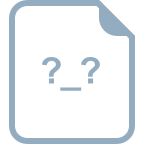
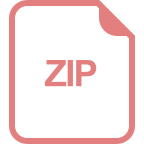
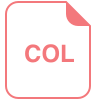












