map<int,int>::iterator t;
时间: 2024-09-10 07:15:53 浏览: 30
在 C++ 中,`map<int, int>::iterator` 是 `std::map` 容器的一个迭代器类型。`std::map` 是一种关联容器,用于存储键值对,其中键(key)是唯一的,值(value)与特定的键关联。当你声明 `t` 类型为 `map<int, int>::iterator` 时,这意味着 `t` 将指向这个 `map` 内部的一对整数(`int` 键和对应的 `int` 值)。
`t` 可以用来遍历整个映射,从第一个元素开始直到最后一个元素。你可以使用 `begin()` 函数获取映射的起始迭代器,用 `end()` 获取结束迭代器(不包括该位置)。例如:
```cpp
std::map<int, int> myMap;
// 添加一些元素到myMap
map<int, int>::iterator t = myMap.begin(); // 初始化迭代器到第一个元素
while (t != myMap.end()) {
std::cout << "Key: " << t->first << ", Value: " << t->second << '\n'; // 输出当前键值对
++t; // 移动到下一个元素
}
```
在这里,`t->first` 访问键(整数),`t->second` 访问对应的值(整数)。
相关问题
map<string,int>::iterator it
`map<string, int>::iterator it` 是C++语言中的一个迭代器声明,用于访问`map<string, int>`类型的容器中的元素。在C++标准库中,`map`是一个关联容器,它存储的元素是键值对,每个元素由一个键(key)和一个值(value)组成,键必须是唯一的。在这个声明中,键是`string`类型,值是`int`类型。
`map<string, int>::iterator`是`map<string, int>`类型的一个迭代器,它提供了一种访问`map`中元素的方式。迭代器类似于指针,但它们更加强大和灵活。使用迭代器可以遍历`map`中的所有元素,进行读取或修改操作。
以下是迭代器的一些基本操作:
1. `it = mymap.begin();`:将迭代器`it`初始化为指向`map`的第一个元素。
2. `it = mymap.end();`:将迭代器`it`设置为指向`map`最后一个元素之后的位置,通常用于循环结束条件。
3. `*it`:解引用迭代器以获取迭代器指向的元素。
4. `it->first`:访问迭代器指向元素的键。
5. `it->second`:访问迭代器指向元素的值。
6. `++it` 或 `it++`:使迭代器前进到下一个元素。
在使用迭代器遍历`map`时,通常采用如下方式:
```cpp
map<string, int> mymap;
// 假设mymap已经被填充了数据
map<string, int>::iterator it;
for (it = mymap.begin(); it != mymap.end(); ++it) {
cout << it->first << " => " << it->second << endl;
}
```
这段代码将输出`mymap`中所有的键值对。
map<string, int>::iterator
An iterator is a pointer-like object that points to an element in a container. In this case, the container is a map of strings to integers.
The iterator is of type `map<string, int>::iterator`, which means it is specifically designed to iterate through the elements of a map with string keys and integer values.
Using this iterator, you can traverse the map, access its elements, and modify them if necessary. For example, you can use the iterator to add a new element to the map, remove an existing element, or update the value of an element.
Here's an example of how to use a `map<string, int>::iterator` to iterate through a map and print out its contents:
```
#include <iostream>
#include <map>
#include <string>
using namespace std;
int main() {
map<string, int> myMap = {{"apple", 3}, {"banana", 2}, {"orange", 5}};
// Iterate through the map using an iterator
for (map<string, int>::iterator it = myMap.begin(); it != myMap.end(); ++it) {
cout << it->first << ": " << it->second << endl;
}
return 0;
}
```
In this example, the `myMap.begin()` function returns an iterator pointing to the first element of the map, and the `myMap.end()` function returns an iterator pointing to the "past-the-end" element of the map.
The `it->first` and `it->second` notation is used to access the key and value of the current element pointed to by the iterator.
The output of this program would be:
```
apple: 3
banana: 2
orange: 5
```
相关推荐
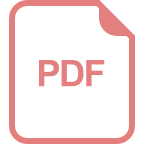
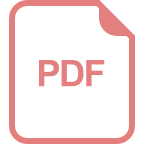
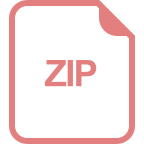












