使用Python的cryptography库 应用AES对“明天中午吃什么”这段文本进行加密解密
时间: 2024-10-15 17:26:43 浏览: 30
在Python中,你可以使用`cryptography`库来应用AES(高级加密标准)对文本进行加密和解密。以下是一个简单的示例,演示如何完成这个过程:
首先,你需要安装`cryptography`库,如果你还没有安装,可以使用pip来安装:
```bash
pip install cryptography
```
然后,以下是使用AES进行加密和解密的步骤:
```python
from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes
from cryptography.hazmat.backends import default_backend
from cryptography.hazmat.primitives.kdf.pbkdf2 import PBKDF2HMAC
# 密码(这里仅用于演示,实际应用中应使用安全的方式获取)
password = b'my_secret_password'
salt = b'some_salt' # 随机生成或者固定值,增加熵
# 使用PBKDF2HMAC生成key
kdf = PBKDF2HMAC(algorithm=algorithms.HMAC(algorithm=algorithms.SHA256(), backend=default_backend()), length=32, salt=salt, iterations=100000)
key = kdf.derive(password)
# 初始化加密模式(CBC是一种常用的模式,适合连续的数据块)
cipher = Cipher(algorithms.AES(key), modes.CBC(key[:16]), backend=default_backend())
# 加密函数
def encrypt(text):
iv = cipher.iv
encryptor = cipher.encryptor()
ct_bytes = encryptor.update(text.encode('utf-8')) + encryptor.finalize()
return iv.hex() + ':' + ct_bytes.hex()
# 解密函数
def decrypt(encrypted_text):
iv, ciphertext = encrypted_text.split(':')
iv = bytes.fromhex(iv)
ciphertext = bytes.fromhex(ciphertext)
decryptor = cipher.decryptor()
pt_bytes = decryptor.update(ciphertext) + decryptor.finalize()
return pt_bytes.decode('utf-8')
# 示例加密和解密
message = "明天中午吃什么"
encrypted_message = encrypt(message)
print(f"Encrypted message: {encrypted_message}")
decrypted_message = decrypt(encrypted_message)
print(f"Decrypted message: {decrypted_message}")
```
在这个例子中,我们使用了密码来计算出AES密钥,并且使用CBC模式进行了加密和解密。注意,在实际生产环境中,你应该存储IV并将其发送给接收方,以便在解密时使用。
阅读全文
相关推荐
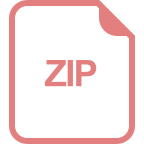
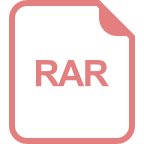
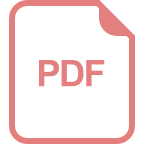















