对回文序列问题,输入一个有n个字符的字符串str[0…n-1],用递归算法编程实现判断str是否为回文串,用C语言
时间: 2024-10-27 14:11:51 浏览: 16
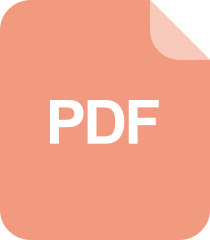
Python针对给定字符串求解所有子序列是否为回文序列的方法
在C语言中,判断一个字符串是否为回文串(正序读和反序读都一样)可以使用递归的方式。基本思路是从字符串的两端开始比较字符,如果相等则继续向中间移动,直到两个指针相遇或者发现不相等的字符为止。以下是递归函数的一个实现:
```c
#include <stdbool.h>
#include <string.h>
bool isPalindrome(char str[], int start, int end) {
// Base cases:
if (start >= end)
return true; // 如果start大于等于end,说明已经检查完一半,都是回文
// 如果首尾字符不相等,则不是回文
if (str[start] != str[end])
return false;
// 否则,继续比较中间部分
return isPalindrome(str, start + 1, end - 1);
}
int main() {
char str[] = "madam";
int length = strlen(str);
if (isPalindrome(str, 0, length - 1))
printf("%s is a palindrome.\n", str);
else
printf("%s is not a palindrome.\n", str);
return 0;
}
```
在这个代码中,`isPalindrome`函数接受三个参数:字符串数组、起始索引和结束索引。当起始索引超过结束索引时,函数返回true,表示字符串是回文;否则,它会检查第一个和最后一个字符是否相同,并递归地检查剩下的部分。
阅读全文
相关推荐
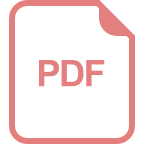
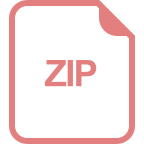



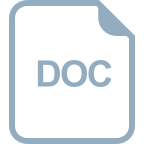
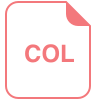
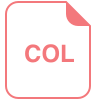
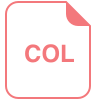
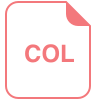
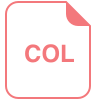
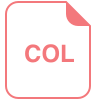
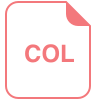
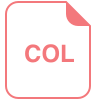
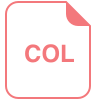
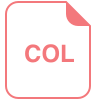
