用C++中栈和队列的基本操作设计用两种以上的算法来实现判断输入的字符串序列是否为回文
时间: 2024-01-22 13:17:38 浏览: 19
算法一:使用栈和队列分别存储字符串序列的前半部分和后半部分,并依次比较它们弹出的字符是否相等。如果全部相等,则该字符串序列为回文。
C++实现:
```c++
#include <iostream>
#include <stack>
#include <queue>
using namespace std;
bool isPalindrome(string str) {
stack<char> s;
queue<char> q;
int len = str.length();
for (int i = 0; i < len/2; i++) {
s.push(str[i]);
}
for (int i = len-1; i >= len/2+(len%2); i--) {
q.push(str[i]);
}
while (!s.empty() && !q.empty()) {
if (s.top() != q.front()) {
return false;
}
s.pop();
q.pop();
}
return true;
}
int main() {
string str = "abba";
if (isPalindrome(str)) {
cout << str << " is a palindrome." << endl;
} else {
cout << str << " is not a palindrome." << endl;
}
return 0;
}
```
算法二:使用递归的方式判断字符串序列是否为回文。将字符序列的首尾字符依次比较,如果相等,则继续比较去掉首尾字符的子串是否为回文,否则该字符串序列不是回文。
C++实现:
```c++
#include <iostream>
using namespace std;
bool isPalindrome(string str) {
int len = str.length();
if (len <= 1) {
return true;
} else {
return str[0] == str[len-1] && isPalindrome(str.substr(1, len-2));
}
}
int main() {
string str = "abba";
if (isPalindrome(str)) {
cout << str << " is a palindrome." << endl;
} else {
cout << str << " is not a palindrome." << endl;
}
return 0;
}
```
相关推荐













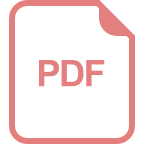
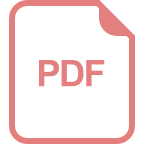