使用C语言,使用栈的算法来实现判断输入的字符串序列是否为回文,写一段完整的程序
时间: 2024-01-22 14:17:40 浏览: 81
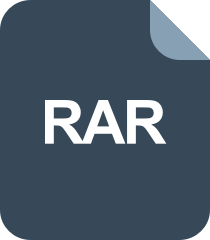
栈判断是否是回文串

下面是使用栈的算法来实现判断字符串序列是否为回文的C语言代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 100 // 栈的最大容量
// 定义栈结构体
typedef struct {
char data[MAX_SIZE];
int top; // 栈顶指针
} Stack;
// 初始化栈
void InitStack(Stack *s) {
s->top = -1;
}
// 进栈
void Push(Stack *s, char ch) {
if (s->top == MAX_SIZE - 1) {
printf("Stack overflow!\n");
exit(1); // 栈满,退出程序
} else {
s->top++;
s->data[s->top] = ch;
}
}
// 出栈
char Pop(Stack *s) {
if (s->top == -1) {
printf("Stack underflow!\n");
exit(1); // 栈空,退出程序
} else {
char ch = s->data[s->top];
s->top--;
return ch;
}
}
// 判断字符串是否为回文
int IsPalindrome(char *s) {
Stack stack;
int len = strlen(s);
int i;
InitStack(&stack);
// 将字符串前一半压入栈中
for (i = 0; i < len / 2; i++) {
Push(&stack, s[i]);
}
// 判断字符串长度的奇偶性
if (len % 2 == 1) {
i++; // 如果是奇数长度,则跳过中间字符
}
// 比较栈中元素和字符串后一半的字符是否相同
while (i < len) {
if (Pop(&stack) != s[i]) {
return 0; // 不是回文
}
i++;
}
return 1; // 是回文
}
int main() {
char s[MAX_SIZE];
printf("请输入字符串:");
scanf("%s", s);
if (IsPalindrome(s)) {
printf("是回文\n");
} else {
printf("不是回文\n");
}
return 0;
}
```
运行程序后,输入一个字符串,程序将会判断该字符串是否为回文。如果是回文,则输出“是回文”,否则输出“不是回文”。
阅读全文
相关推荐



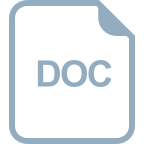











