队列完成二进制、十进制的相互转换的C语言代码
时间: 2024-10-12 10:13:24 浏览: 28
队列可以用于辅助二进制和十进制之间的转换,因为它支持先进先出(FIFO)的数据结构特性,适合逐步处理每一位数字。以下是简单的C语言代码示例,使用数组模拟队列,将十进制数转换成二进制:
```c
#include <stdio.h>
#include <stdlib.h>
#define QUEUE_SIZE 10
// 创建空队列
int queue[QUEUE_SIZE] = {0};
int front = -1, rear = -1;
// 向队列添加数字
void enqueue(int num) {
if ((rear + 1) % QUEUE_SIZE == front) {
printf("Queue is full.\n");
return;
}
rear = (rear + 1) % QUEUE_SIZE;
queue[rear] = num;
}
// 从队列移除数字并返回
int dequeue() {
if (front == -1) {
printf("Queue is empty.\n");
return -1;
}
int temp = queue[front];
front = (front + 1) % QUEUE_SIZE;
return temp;
}
// 十进制转二进制
void decimal_to_binary(int decimal) {
while (decimal > 0) {
enqueue(decimal % 2);
decimal /= 2;
}
// 反向遍历队列打印结果
while (front != -1) {
printf("%d", dequeue());
}
printf("\n");
}
// 示例
int main() {
int decimal_num = 42;
printf("Decimal to Binary: ");
decimal_to_binary(decimal_num);
return 0;
}
```
对于从二进制到十进制的转换,你可以反向处理队列,每次取出一位乘以2的相应次方,然后累加。注意,这个简单版本只适用于比较小的数值,因为它的内存限制了队列的大小。
阅读全文
相关推荐
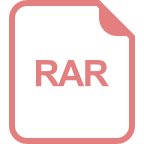
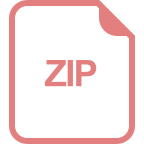
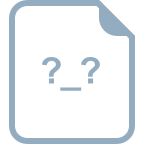


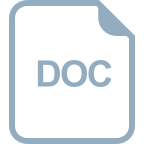
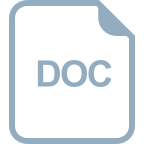






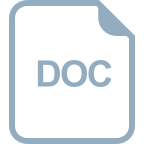
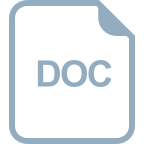
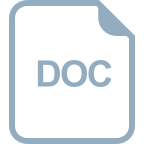
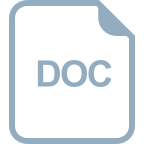
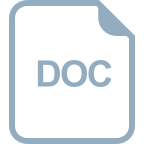
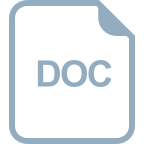