选择DFS、BFS、A算法或A*算法中的一种来解决八数码问题。将初始状态转换为最终状态。(注:代码要添加注释)
时间: 2024-09-27 12:03:15 浏览: 31
在解决八数码问题(也称为数独游戏)时,A*算法通常是最合适的选择,因为它是一种启发式搜索算法,结合了广度优先搜索(BFS)的优点(保证找到最短路径)以及深度优先搜索(DFS)的部分特征(探索更深层节点)。以下是使用Python实现A*算法的一个简化版示例:
```python
# 定义节点类,包含状态值、代价和前驱节点
class Node:
def __init__(self, state, cost=0, prev=None):
self.state = state
self.cost = cost
self.prev = prev
# A*搜索函数
def a_star(start_state, goal_state, heuristic_func):
open_set = [Node(start_state, 0)]
closed_set = []
while open_set:
current_node = min(open_set, key=lambda x: x.cost + heuristic_func(x.state, goal_state))
# 如果当前节点是目标状态,返回解决方案路径
if current_node.state == goal_state:
path = []
while current_node:
path.append(current_node.state)
current_node = current_node.prev
return path[::-1] # 返回路径从目标到起点
open_set.remove(current_node)
closed_set.append(current_node)
for neighbor in generate_neighbors(current_node.state): # 生成相邻合法状态
new_cost = current_node.cost + 1 # 更新代价
if is_valid_neighbor(neighbor, closed_set): # 检查邻居是否有效并未被访问过
if neighbor not in open_set:
open_set.append(Node(neighbor, new_cost, current_node))
elif new_cost < open_set[open_set.index(neighbor)].cost:
open_set[open_set.index(neighbor)] = Node(neighbor, new_cost, current_node)
# 假设generate_neighbors()和is_valid_neighbor()分别用于生成状态的邻接点和验证有效性
```
在这个例子中,`heuristic_func`是一个估算从当前节点到目标节点成本的函数,常见的比如曼哈顿距离等。A*算法会在每次迭代时优先处理估计代价最低的节点。
阅读全文
相关推荐
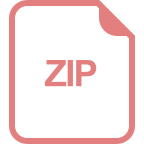
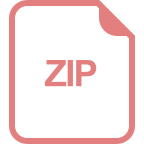
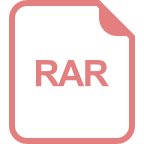
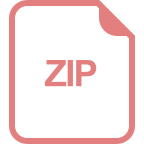
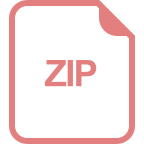
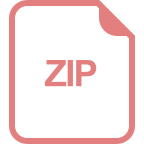
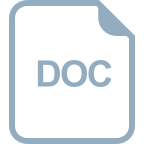
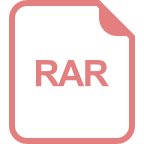
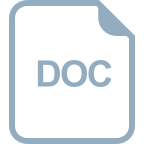
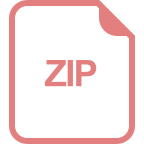
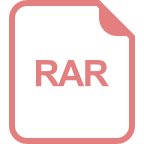
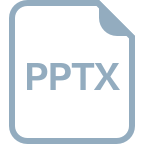
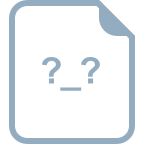
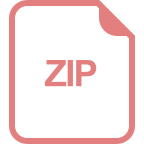
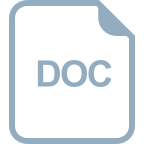
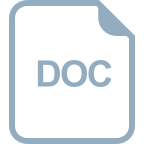
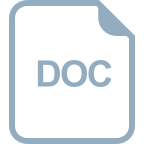

