用c++编写用深度优先算法和广度优先算法解决八数码问题,并且定义初始状态和随机状态
时间: 2024-02-11 22:04:49 浏览: 22
好的,我可以给你提供一个样例代码,你可以参考一下。这里我们使用结构体 `State` 来表示八数码问题中的状态,其中 `grid` 表示方格中的数字,`x` 和 `y` 表示空格的位置。
```cpp
#include <iostream>
#include <cstring>
#include <algorithm>
#include <queue>
#include <set>
using namespace std;
const int N = 3;
struct State {
int grid[N][N];
int x, y;
bool operator==(const State& other) const {
return memcmp(grid, other.grid, sizeof(grid)) == 0;
}
};
State target_state = {
{{1, 2, 3}, {4, 5, 6}, {7, 8, 0}},
2, 2
};
void print_state(const State& state) {
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
cout << state.grid[i][j] << " ";
}
cout << endl;
}
cout << endl;
}
State move(const State& state, int dir) {
State next_state = state;
int dx[] = {-1, 0, 1, 0}, dy[] = {0, 1, 0, -1};
int nx = state.x + dx[dir], ny = state.y + dy[dir];
if (nx < 0 || nx >= N || ny < 0 || ny >= N) {
return state;
}
swap(next_state.grid[state.x][state.y], next_state.grid[nx][ny]);
next_state.x = nx;
next_state.y = ny;
return next_state;
}
void dfs(State state, int depth, int max_depth) {
if (state == target_state) {
print_state(state);
exit(0);
}
if (depth > max_depth) {
return;
}
for (int i = 0; i < 4; i++) {
State next_state = move(state, i);
if (next_state != state) {
dfs(next_state, depth + 1, max_depth);
}
}
}
void bfs(State start_state) {
queue<State> q;
set<State> visited;
q.push(start_state);
visited.insert(start_state);
while (!q.empty()) {
State state = q.front();
q.pop();
if (state == target_state) {
print_state(state);
exit(0);
}
for (int i = 0; i < 4; i++) {
State next_state = move(state, i);
if (next_state != state && visited.count(next_state) == 0) {
q.push(next_state);
visited.insert(next_state);
}
}
}
}
State random_state() {
State state;
vector<int> nums = {1, 2, 3, 4, 5, 6, 7, 8, 0};
random_shuffle(nums.begin(), nums.end());
for (int i = 0; i < 9; i++) {
state.grid[i / 3][i % 3] = nums[i];
if (nums[i] == 0) {
state.x = i / 3;
state.y = i % 3;
}
}
return state;
}
int main() {
State start_state = random_state();
cout << "Start state:" << endl;
print_state(start_state);
cout << "Target state:" << endl;
print_state(target_state);
cout << "DFS result:" << endl;
for (int i = 1; i <= 100; i++) {
dfs(start_state, 0, i);
}
cout << "BFS result:" << endl;
bfs(start_state);
return 0;
}
```
在主函数中,我们首先使用 `random_state` 函数来随机生成初始状态,并且输出初始状态和目标状态。接下来,我们使用深度优先算法和广度优先算法来分别搜索这个问题的解,输出结果即可。
需要注意的是,在深度优先算法中,我们需要设置最大搜索深度,因为如果没有最大搜索深度,程序可能会陷入死循环。在本代码中,我们设置最大搜索深度为 $100$,如果搜索深度超过 $100$,则返回上一个状态。同时,为了提高搜索效率,我们可以加入一些剪枝操作。在广度优先算法中,我们使用 `set` 来记录已经访问过的状态,以避免重复访问和死循环。
希望这个样例代码可以帮助到你。
相关推荐
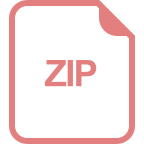
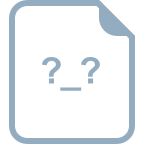
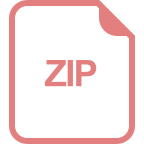














