python制作一个可用广度优先算法,深度优先算法和A*算法解决九宫重排问题,要求自己输入初始状态和最终状态
时间: 2023-11-18 22:09:06 浏览: 81
好的,我可以帮你实现这个问题。首先,为了方便,我们将九宫重排问题抽象为一个简单的矩阵,通过交换空格和数字来达到目标状态。
接下来,我们分别介绍三种算法的实现方法。
## 广度优先搜索算法
广度优先搜索算法(BFS)从初始状态开始,逐层扩展,直到找到目标状态。我们可以用队列来实现BFS。
``` python
def bfs(start, end):
queue = [(start, [])]
visited = set([start])
while queue:
(node, path) = queue.pop(0)
if node == end:
return path
for move, action in next_moves(node):
next_node = apply_move(node, move)
if next_node not in visited:
queue.append((next_node, path + [action]))
visited.add(next_node)
return None
```
其中,`start`和`end`分别是初始状态和目标状态,`next_moves`函数返回当前状态下所有可行的移动方案,`apply_move`函数返回应用指定移动方案后的新状态。
## 深度优先搜索算法
深度优先搜索算法(DFS)是一种递归算法,它从初始状态开始,尽可能深地搜索,直到找到目标状态或无法继续搜索为止。
``` python
def dfs(start, end, depth):
if depth == 0:
return None
if start == end:
return []
for move, action in next_moves(start):
next_node = apply_move(start, move)
if next_node not in visited:
visited.add(next_node)
path = dfs(next_node, end, depth - 1)
if path is not None:
return [action] + path
return None
```
其中,`depth`参数表示当前搜索的深度,`visited`集合用于记录已访问过的状态。
## A*搜索算法
A*搜索算法是一种启发式搜索算法,它通过估计当前状态到目标状态的距离来指导搜索方向。我们可以使用曼哈顿距离作为估价函数。
``` python
def heuristic(state, end):
distance = 0
for i in range(3):
for j in range(3):
if state[i][j] != 0:
x, y = divmod(end.index(state[i][j]), 3)
distance += abs(i - x) + abs(j - y)
return distance
def astar(start, end):
queue = [(heuristic(start, end), start, [])]
visited = set([start])
while queue:
(_, node, path) = heapq.heappop(queue)
if node == end:
return path
for move, action in next_moves(node):
next_node = apply_move(node, move)
if next_node not in visited:
visited.add(next_node)
heapq.heappush(queue, (heuristic(next_node, end) + len(path) + 1, next_node, path + [action]))
return None
```
其中,`heuristic`函数返回当前状态到目标状态的估计距离,`heapq`模块用于按优先级排序队列。
下面是完整的代码实现:
相关推荐
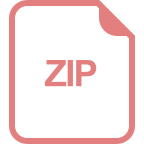
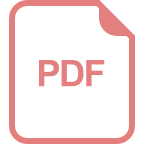
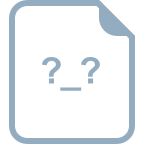














