python,利用牛顿迭代法求出1到n之间的所有整数的算术平方根,并与math库中的sqrt 函数的结果比较
时间: 2024-10-06 15:03:32 浏览: 48
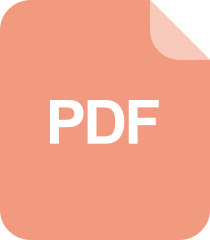
Python编程实现二分法和牛顿迭代法求平方根代码
在Python中,你可以使用牛顿迭代法(Newton-Raphson Method)来近似计算一个数的平方根,这是一种迭代优化算法。对于每个数`x`,我们从一个初始猜测值开始,比如`x / 2`,然后不断逼近真实值直到满足精度要求。以下是实现步骤:
```python
import math
def newton_sqrt(n, tolerance=1e-8):
guess = n
while abs(guess * guess - n) > tolerance:
guess = (guess + n / guess) / 2
return guess
# 获取1到n的所有整数并计算它们的算术平方根
for i in range(1, n+1):
approx_root = newton_sqrt(i)
exact_root = math.sqrt(i)
print(f"Approximate square root of {i} is {approx_root}, and the exact is {exact_root}")
# 比较两者结果
print("Comparison:")
for i, (approx, exact) in enumerate(zip(approx_root, exact_root)):
if approx == exact:
print(f"{i}: Both methods agree for sqrt({i})")
else:
print(f"{i}: Newton's method gives {approx}, whereas math.sqrt gives {exact}")
```
在这个例子中,我们将每个数字的近似平方根与`math.sqrt()`库函数的结果进行对比。请注意,牛顿迭代法虽然通常收敛很快,但对于非常大的数值可能会有性能问题,因为它涉及大量的除法操作。
阅读全文
相关推荐
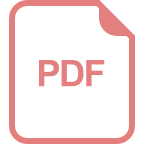
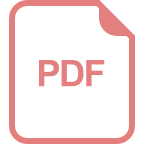






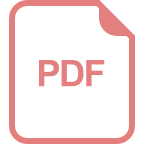
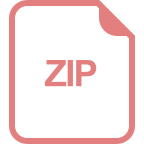
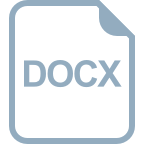
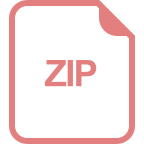
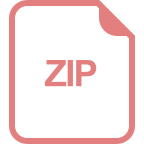
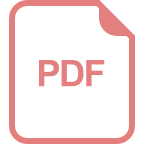
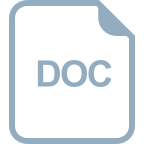
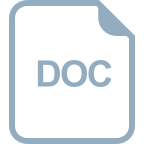