用OpenGL编写程序,根据用户鼠标单击位置确定的3个顶点绘制三角形;鼠标右击完成三角形的平移。单击三次鼠标左键后画出白色三角形,单击右键将三角形的中心移动至该地方。(不要省略,给出完整的三角形平移逻辑的代码!)
时间: 2024-11-30 20:21:55 浏览: 9
首先,我们需要设置一个OpenGL环境并处理鼠标的输入事件。以下是一个基本的示例,展示了如何使用GLFW库进行窗口创建、渲染三角形以及处理鼠标事件:
```cpp
#include <GLFW/glfw3.h>
#include <iostream>
class Triangle {
public:
float vertices[3][3] = {{0, 0, 0}, {1, 0, 0}, {0.5, sqrt(3)/2, 0}}; // 默认三角形的位置
float translateX, translateY;
void draw() {
glBegin(GL_TRIANGLES);
glVertex3f(vertices[0][0], vertices[0][1], vertices[0][2]);
glVertex3f(vertices[1][0], vertices[1][1], vertices[1][2]);
glVertex3f(vertices[2][0], vertices[2][1], vertices[2][2]);
glEnd();
}
void onRightClick(int x, int y) {
translateX += x - glfwGetCursorPos(window, nullptr)[0];
translateY += y - glfwGetCursorPos(window, nullptr)[1];
for (int i = 0; i < 3; ++i) {
vertices[i][0] += translateX;
vertices[i][1] += translateY;
}
}
private:
GLFWwindow* window;
};
int main() {
if (!glfwInit()) {
std::cerr << "Failed to initialize GLFW" << std::endl;
return -1;
}
// 创建窗口
window = glfwCreateWindow(800, 600, "Triangle Example", NULL, NULL);
if (!window) {
glfwTerminate();
return -1;
}
// 设置回调函数
glfwSetMouseButtonCallback(window, mouseButtonCallback);
glfwSetCursorPosCallback(window, cursorPosCallback);
// 初始化OpenGL上下文
glfwMakeContextCurrent(window);
glClearColor(0.2f, 0.3f, 0.3f, 1.0f);
glEnable(GL_DEPTH_TEST);
Triangle triangle;
while (!glfwWindowShouldClose(window)) {
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
triangle.draw();
// 右键点击事件
if (glfwGetMouseButton(window, GLFW_MOUSE_BUTTON_RIGHT) == GLFW_PRESS) {
triangle.onRightClick(glfwGetCursorPos(window, nullptr)[0], glfwGetCursorPos(window, nullptr)[1]);
}
glfwSwapBuffers(window);
glfwPollEvents();
}
glfwTerminate();
return 0;
}
void mouseButtonCallback(GLFWwindow* window, int button, int action, int mods) {
if (button == GLFW_MOUSE_BUTTON_LEFT && action == GLFW_PRESS) {
// 保存三角形顶点以便于后续绘制
// 这里假设你已经记录了当前的鼠标位置
} else if (button == GLFW_MOUSE_BUTTON_RIGHT && action == GLFW_PRESS) {
// 右键点击事件处理
}
}
void cursorPosCallback(GLFWwindow* window, double xpos, double ypos) {
// 更新鼠标位置
}
```
这段代码演示了如何在OpenGL环境中响应鼠标点击和右击事件。请注意,实际项目中你需要添加额外的逻辑来存储和处理鼠标点击位置,以及在`mouseButtonCallback`函数中执行相应的三角形顶点更新操作。
阅读全文
相关推荐
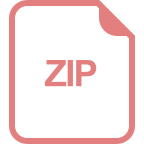
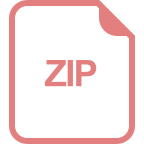





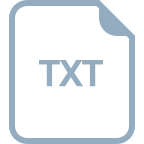
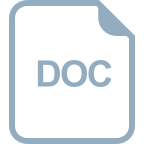
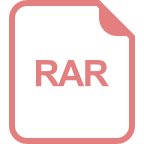
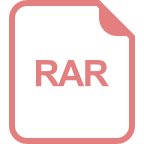
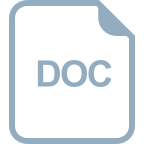
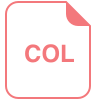
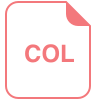

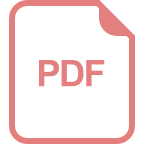
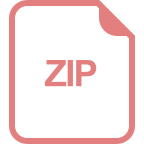
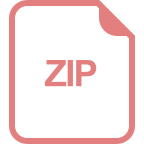