差分进化算法python代码
时间: 2023-11-09 21:03:33 浏览: 253
差分进化算法(Differential Evolution,DE)是一种常用的全局优化算法,适用于连续优化问题。下面是一个简单的差分进化算法的 Python 代码实现:
```python
import random
import numpy as np
def differential_evolution(fobj, bounds, popsize=20, mut=0.8, crossp=0.7, maxiter=100):
"""
Differential evolution optimization algorithm.
Parameters:
-----------
fobj : function
The objective function to be minimized.
bounds : list of tuples
The bounds of the design variables.
popsize : int, optional
The population size. Default is 20.
mut : float, optional
The mutation factor. Default is 0.8.
crossp : float, optional
The crossover probability. Default is 0.7.
maxiter : int, optional
The maximum number of iterations. Default is 100.
Returns:
--------
best : array_like
The best solution found.
"""
# Initialize the population randomly within the bounds
pop = np.random.rand(popsize, len(bounds))
min_b, max_b = np.asarray(bounds).T
diff = np.fabs(min_b - max_b)
pop_denorm = min_b + pop * diff
# Evaluate the initial population
fitness = np.asarray([fobj(ind) for ind in pop_denorm])
# Find the best individual and its fitness value
best_idx = np.argmin(fitness)
best = pop_denorm[best_idx]
# Iterate until the maximum number of iterations is reached
for i in range(maxiter):
for j in range(popsize):
# Select three random individuals, excluding the current one
idxs = [idx for idx in range(popsize) if idx != j]
a, b, c = pop[np.random.choice(idxs, 3, replace=False)]
# Mutation
mutant = np.clip(a + mut * (b - c), 0, 1)
# Crossover
cross_points = np.random.rand(len(bounds)) < crossp
if not np.any(cross_points):
cross_points[np.random.randint(0, len(bounds))] = True
trial = np.where(cross_points, mutant, pop[j])
# Denormalize trial solution
trial_denorm = min_b + trial * diff
# Evaluate trial solution
f = fobj(trial_denorm)
# Update the population if the trial solution is better
if f < fitness[j]:
fitness[j] = f
pop[j] = trial
if f < fitness[best_idx]:
best_idx = j
best = trial_denorm
# Print the current best fitness value every 10 iterations
if i % 10 == 0:
print("Iteration {}: Best fitness value = {}".format(i, fitness[best_idx]))
return best
```
其中,fobj 是目标函数,bounds 是设计变量的上下界,popsize 是种群大小,mut 是变异因子,crossp 是交叉概率,maxiter 是最大迭代次数。函数返回最优解。
阅读全文
相关推荐
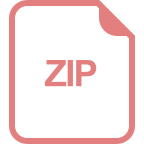
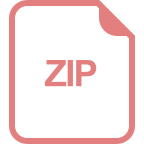
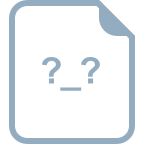











