用C写 76byte以太网数据转换CAN报文
时间: 2024-09-23 15:13:18 浏览: 43
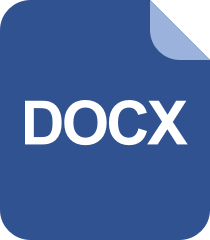
S7-1200中将BYTE类型数据转换成char类型数据的具体方法.docx
在C语言中,将Ethernet(以太网)数据转换成CAN(控制器局域网络)报文通常涉及到几个步骤,因为它们的数据结构和协议差异很大。由于你提到的是76字节的数据长度,这里是一个简化的示例,假设你已经有了一个以太网数据包头和有效负载,并且需要提取前部分用于CAN报文:
```c
#include <stdio.h>
#include <stdint.h>
// Ethernet header (20 bytes)
struct EthernetHeader {
uint8_t dstMAC[ETH_ALEN];
uint8_t srcMAC[ETH_ALEN];
uint16_t ethType;
};
// CAN data structure (8 bytes for ID + payload)
struct CANData {
uint32_t canID; // CAN identifier
uint8_t payload[6]; // Up to 6 bytes of useful data from Ethernet
};
void ethToCan(const struct EthernetHeader *eth, struct CANData *can) {
// Assuming eth->ethType is the start of the useful data in the Ethernet packet
uint8_t useful_data[7] = {eth->ethType, /* Followed by additional 5 bytes from Ethernet*/ };
// Copy the first 6 bytes to CAN payload
memcpy(can->payload, useful_data, sizeof(can->payload));
// Set CAN ID, assuming it's a fixed offset from the Ethernet packet
can->canID = *(uint32_t*)(eth + 1); // Assuming the ID starts at the next byte after MAC addresses
printf("Converted CAN message:\n");
printf("ID: %08X\n", can->canID);
printf("Payload: ");
for (int i = 0; i < 6; i++) {
printf("%02X ", can->payload[i]);
}
}
int main() {
// Replace with actual Ethernet data here
struct EthernetHeader eth;
// ... fill in the Ethernet header fields ...
struct CANData can;
ethToCan(ð, &can);
return 0;
}
```
这个示例仅适用于简化场景,实际应用中可能需要处理更复杂的帧结构、错误检测以及填充等。记住,在真实环境中,你需要根据网络协议规范来设计和处理数据。
阅读全文
相关推荐
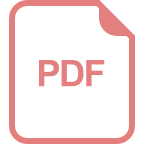
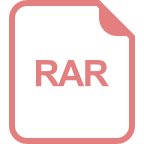


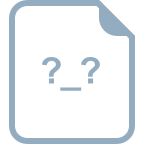











