如何在Python 2.7和Python 3.0中实现LZW算法,并对压缩效果进行评估?请提供可运行的代码示例。
时间: 2024-12-02 07:24:57 浏览: 0
LZW算法是一种经典的无损数据压缩算法,适用于处理具有重复模式的文本数据。在Python中实现LZW算法,需要编写函数来处理字符串的读取、字典表的动态构建和管理,以及编码和解码的过程。以下是一个简化的LZW算法实现示例代码,该代码兼容Python 2.7和Python 3.0,并提供了一个评估压缩效果的方法:
参考资源链接:[LZW压缩算法Python实现及运行示例](https://wenku.csdn.net/doc/5zi54uymo2?spm=1055.2569.3001.10343)
```python
def create_lzw_dictionary(input_string):
dictionary = {chr(i): [chr(i)] for i in range(256)}
dictionary[''.join([chr(i), chr(i)]): [chr(i), chr(i)] for i in range(256, 512)}
for i in range(512, 768):
dictionary[''.join([chr(i-256), chr(i-256)]): [chr(i-256), chr(i-256)]}
return dictionary
def compress(input_string):
dictionary = create_lzw_dictionary(input_string)
current_string = input_string[0]
compressed_output = []
for i in range(1, len(input_string)):
next_string = input_string[i]
if current_string + next_string in dictionary:
current_string = current_string + next_string
else:
compressed_output.append(dictionay[current_string])
dictionary[current_string + next_string] = dictionary.get(current_string,[]) + [next_string]
current_string = next_string
compressed_output.append(dictionary[current_string])
return compressed_output
def decompress(compressed_output, dictionary):
decompressed_output = []
for code in compressed_output:
if code == dictionary:
break
current_code = code
decompressed_output.append(code[0])
for i in range(len(code)):
if code[i] in dictionary.keys():
break
decompressed_output.append(code[i])
dictionary = {v:[k] + v for k, v in dictionary.items() if k[0] == code[i]}
return ''.join(decompressed_output)
def evaluate_compression(input_string):
compressed = compress(input_string)
compressed_size = len(''.join(compressed))
decompressed = decompress(compressed, create_lzw_dictionary(input_string))
assert input_string == decompressed
decompressed_size = len(input_string)
compression_ratio = float(compressed_size) / decompressed_size
return compression_ratio
# 示例使用
input_string = 'TOBEORNOTTOBEORTOBEORNOT#'
compression_ratio = evaluate_compression(input_string)
print(f'Compression Ratio: {compression_ratio}')
```
这段代码首先定义了创建初始字典的函数`create_lzw_dictionary`,然后是压缩函数`compress`和解压函数`decompress`。通过调用`evaluate_compression`函数,可以得到压缩比例来评估压缩效果。
在实际应用中,为了达到更好的压缩效果和处理更复杂的数据类型,LZW算法实现可能需要进行优化,包括但不限于优化字典表的管理、处理特殊字符、以及优化算法的执行效率。
建议读者在理解上述代码后,查阅《LZW压缩算法Python实现及运行示例》以获取更深入的理解和实现更复杂的场景。这份资料详细介绍了LZW算法的原理和在Python中的实现,包含了代码示例和运行指南,能够帮助读者全面掌握LZW算法及其在Python中的应用。
参考资源链接:[LZW压缩算法Python实现及运行示例](https://wenku.csdn.net/doc/5zi54uymo2?spm=1055.2569.3001.10343)
阅读全文
相关推荐
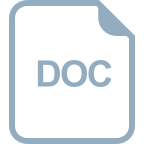
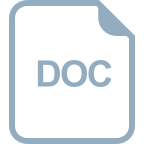
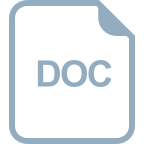
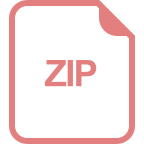
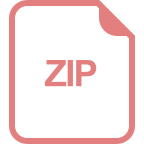
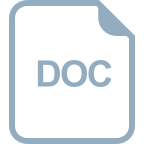
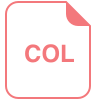
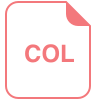










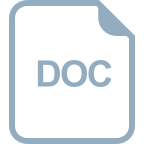