给定公元1000年到公元9999年之间的某一天,给出该天的后天是哪一天
时间: 2024-09-23 22:12:21 浏览: 79
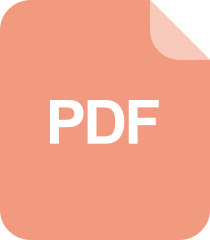
Python编程实现输入某年某月某日计算出这一天是该年第几天的方法

要计算公元1000年到公元9999年之间任意一天后的第二天,你可以创建一个程序来处理这个逻辑。首先,你需要考虑闰年的规则,因为每四年有一个闰年,但是能被100整除但不能被400整除的年份不是闰年。以下是一个简单的C语言函数,用于找到指定日期后的第二天:
```c
#include <stdio.h>
// 辅助函数检查是否为闰年
int is_leap_year(int year) {
return (year % 4 == 0 && (year % 100 != 0 || year % 400 == 0));
}
// 计算日期
void calculate_day_after_two_days(int year, int month, int day) {
// 检查月份范围(不包括2月)
if (month < 1 || month > 12) {
printf("Invalid month.\n");
return;
}
// 修正2月份的天数(根据闰年和平年)
int days_in_month[] = {31, 28 + is_leap_year(year), 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
day += 2;
// 如果加上两天超过当月天数,加上一年并减去剩余月份的第一天
if (day > days_in_month[month - 1]) {
day -= days_in_month[month - 1];
year++;
month = 1; // 跳过当前月,从下一个月开始
} else {
month++;
}
// 输出结果
printf("The day after two days from %d-%02d-%02d is %d-%02d-%02d.\n",
year, month, day, year, month, day);
}
int main() {
int year, month, day;
printf("Enter a date between 1000 and 9999 in the format YYYY-MM-DD: ");
scanf("%d-%d-%d", &year, &month, &day);
// 检查日期是否在指定范围内
if (year >= 1000 && year <= 9999 && month >= 1 && month <= 12 && day >= 1 && day <= 31) {
calculate_day_after_two_days(year, month, day);
} else {
printf("Date out of range.\n");
}
return 0;
}
```
当你运行此程序时,它会提示用户输入一个日期,然后输出指定日期的后两天。
阅读全文
相关推荐
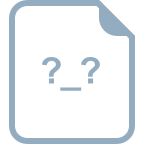

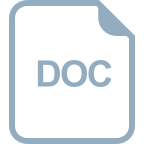
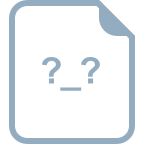












