用c语言计算输入的某一天是该年的第几天
时间: 2024-11-06 09:16:48 浏览: 29
在C语言中,计算给定日期是一年中的第几天,需要考虑闰年的情况。我们可以使用蔡勒公式(Zeller's congruence)来简化计算过程,该公式适用于从公元1到9999的所有公历日期。以下是基本步骤:
1. 获取输入的月份(注意是0-based,即1月为0),例如如果是3月,输入为2。
2. 获取输入的日,例如15日。
3. 获取输入的年份,可以是公元纪年。
4. 应对特殊情况:如果输入的年份能被100整除但不是世纪闰年,则不是闰年;如果能被400整除则是世纪闰年。
5. 根据蔡勒公式计算结果:
```cpp
int dayOfYear(int month, int day, int year) {
int q = day;
int m = month + 1; // 转换为0-based
int K = year % 100;
int J = year / 100;
int h = (q + ((13 * (m + 1)) / 5) + K + (K / 4) + (J / 4) - (2 * J)) % 7;
// 计算实际天数
int days = (h == 6 || h == 0) ? 31 : (h == 2) ? 28 : (year % 4 == 0 && (year % 100 != 0 || year % 400 == 0)) ? 29 : 28;
return (m > 2) ? (days + sumDaysBefore(m, year)) : days;
}
// 计算前几个月的总天数(闰年2月29天)
int sumDaysBefore(int month, int year) {
int total = 0;
switch(month) {
case 1: total += 31; break;
case 2: if (isLeapYear(year)) total += 29; else total += 28; break;
default: total += month * 30; break;
}
return total;
}
bool isLeapYear(int year) {
return (year % 4 == 0 && (year % 100 != 0 || year % 400 == 0));
}
int main() {
int month, day, year;
printf("Enter the month (0-11), day, and year: ");
scanf("%d %d %d", &month, &day, &year);
int result = dayOfYear(month, day, year);
printf("The given date (%d/%d/%d) is the %dth day of the year.\n", day, month + 1, year, result);
return 0;
}
```
阅读全文
相关推荐
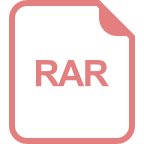















