任务:编写一个程序,接受用户输入的文本,并分析以下内容: 提取所有的单词,并统计每个单词的出现次数 查找并提取所有的价格(格式为¥XX.XX) 替换所有的数子为#符号 要求: 输出每个单词及其出现的次数 输出提取的所有价格 输出替换后的文本》
时间: 2024-10-16 22:05:15 浏览: 27
为了编写这个程序,你可以使用Python语言,因为其丰富的库支持字符串操作和数据分析。这里是一个简单的步骤概述:
1. **获取用户输入**:
使用`input()`函数获取用户的文本输入。
2. **单词提取和计数**:
- 使用`split()`函数将文本分割成单词列表。
- 创建一个空字典`word_counts`用于存储单词及其出现次数。
- 遍历单词列表,更新字典中的计数。
```python
text = input()
words = text.split()
word_counts = {word: words.count(word) for word in words}
```
3. **价格查找**:
使用正则表达式匹配价格模式。假设价格格式为“¥XX.XX”,可以使用`re.findall()`函数。
```python
import re
prices = re.findall(r'¥\d+\.\d+', text)
```
4. **替换数字**:
使用`str.replace()`函数将所有数字替换为`#`。
```python
def replace_numbers(text):
return ''.join('#' if ch.isdigit() else ch for ch in text)
modified_text = replace_numbers(text)
```
5. **输出结果**:
最后,分别打印出单词计数、价格列表以及替换后的文本。
```python
for word, count in word_counts.items():
print(f"{word}: {count}")
print("\nPrices found:")
for price in prices:
print(price)
print("\nModified text:")
print(modified_text)
```
阅读全文
相关推荐
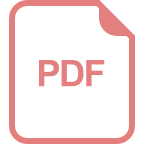
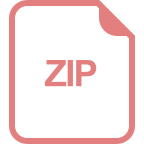
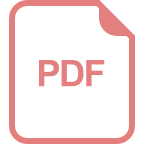















