如何使用C语言实现泰勒级数法来计算正弦函数sin(x)?
时间: 2024-10-29 22:19:20 浏览: 137
使用C语言实现泰勒级数法来计算正弦函数sin(x),通常涉及到编写一个递归或循环结构来逐步近似正弦值。泰勒级数展开式是:
sin(x) = x - (x^3 / 3!) + (x^5 / 5!) - ... + (-1)^(n+1) * (x^(2n+1) / (2n+1)!)
以下是一个简单的C程序示例,展示了如何使用前几项进行计算:
```c
#include <stdio.h>
#include <math.h>
double factorial(int n) {
if (n == 0 || n == 1) return 1;
else return n * factorial(n - 1);
}
double sin_taylor(double x, int terms) {
double sum = x, fact = factorial(3), term = 1.0;
for (int i = 2; i <= terms && abs(term) > 1e-8; ++i) {
term *= -1 * pow(x, 2 * i + 1);
fact /= (2 * i + 1);
sum += term / fact;
}
return sum;
}
int main() {
double x, result;
printf("Enter the value of x: ");
scanf("%lf", &x);
int terms = 10; // 可以调整需要的精度,更多的项会得到更准确的结果
result = sin_taylor(x, terms);
printf("The approximation of sin(%lf) using Taylor series with %d terms is: %lf\n", x, terms, result);
return 0;
}
```
这个程序首先定义了一个计算阶乘的辅助函数,然后在`sin_taylor`函数中迭代地添加泰勒级数的每一项。用户可以输入他们想要计算的`x`值以及希望取多少项来进行近似。
阅读全文
相关推荐
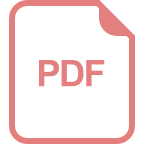
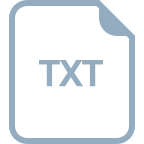
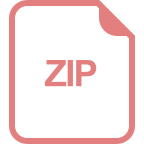















