.房间内有一只猴子,若干个箱子,猴子身高50cm,箱子高50cm,天花板上挂的香蕉离地面150cm,请编写C语言程序完成猴子摘香蕉的问题
时间: 2024-11-03 12:13:14 浏览: 11
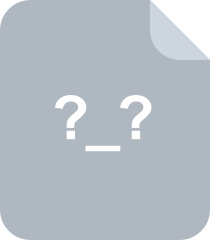
猴子摘香蕉问题求解完整代码
在C语言中,为了模拟这个场景,我们可以创建一个简单的控制台游戏或函数,假设我们只有基本的条件判断功能。由于猴子无法直接跳到天花板上,我们可以通过让猴子尝试拿起地面上的箱子叠加起来试图触碰香蕉。这里是一个简化的示例:
```c
#include <stdio.h>
// 定义猴子、箱子和香蕉的结构体
typedef struct {
int height;
} Monkey, Box, Banana;
// 检查猴子是否能拿箱子
int can_pick(Box box, Monkey monkey) {
return box.height >= monkey.height;
}
// 让猴子叠箱子
void stack_boxes(Box* boxes, int num_boxes, Monkey monkey) {
for (int i = 0; i < num_boxes - 1; ++i) {
if (can_pick(boxes[i + 1], monkey)) {
monkey.height += boxes[i].height;
} else {
break; // 如果猴子拿不起下一个箱子,则停止叠加
}
}
}
// 主函数
int main() {
Monkey monkey;
monkey.height = 50; // 猴子初始高度
Box boxes[] = { {50}, {50}, {50} }; // 假设有三个等高的箱子
int num_boxes = sizeof(boxes) / sizeof(Box);
// 尝试拿箱子,如果可以,一直叠加直到最大高度
stack_boxes(boxes, num_boxes, monkey);
// 判断猴子能否摸到香蕉
if (monkey.height >= 150) {
printf("Monkey can reach the banana by stacking boxes.\n");
} else {
printf("Monkey cannot reach the banana with the given boxes.\n");
}
return 0;
}
```
注意这只是一个非常基础的示例,并未涉及复杂的游戏循环或输入验证。此外,在实际应用中,更完善的程序会考虑到猴子每次只能拿一个箱子以及如何处理错误情况。
阅读全文
相关推荐
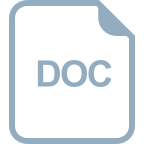
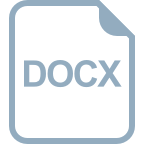











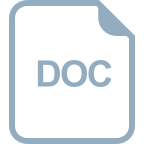
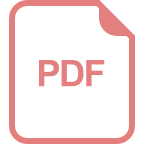
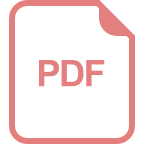
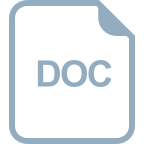
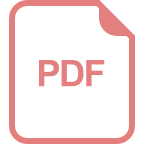