compare_map_filter
时间: 2023-08-13 10:04:44 浏览: 122
`map`, `filter`, and `reduce` are three higher-order functions commonly used in functional programming languages like JavaScript, Python, and others. These functions operate on lists, arrays, or other iterable objects and allow for concise and expressive manipulation of data.
Here's a brief explanation of each function:
1. `map`: The `map` function applies a given function to each element in a list and returns a new list with the transformed values. It takes in two arguments: the function to apply and the list to operate on. The resulting list will have the same length as the original list.
Example:
```python
# Double each element in the list using map
numbers = [1, 2, 3, 4, 5]
doubled_numbers = list(map(lambda x: x * 2, numbers))
print(doubled_numbers) # Output: [2, 4, 6, 8, 10]
```
2. `filter`: The `filter` function creates a new list by selecting elements from an existing list that satisfy a given condition. It takes in two arguments: the condition (often expressed as a lambda function) and the list to filter. Only the elements that evaluate to `True` for the condition will be included in the resulting list.
Example:
```python
# Filter out even numbers from the list using filter
numbers = [1, 2, 3, 4, 5]
filtered_numbers = list(filter(lambda x: x % 2 == 0, numbers))
print(filtered_numbers) # Output: [2, 4]
```
3. `reduce`: The `reduce` function applies a given function to a sequence of elements, reducing them to a single value. It takes in two arguments: the function to apply and the sequence to reduce. The function must take two arguments and return a single value. The result of each reduction is passed as the first argument to the next reduction until a single value is obtained.
Example:
```python
from functools import reduce
# Compute the sum of all elements in the list using reduce
numbers = [1, 2, 3, 4, 5]
sum_of_numbers = reduce(lambda x, y: x + y, numbers)
print(sum_of_numbers) # Output: 15
```
In summary, `map` allows for transforming each element in a list, `filter` allows for selecting elements based on a condition, and `reduce` allows for combining elements into a single value. These functions provide powerful tools for manipulating and processing data in a functional programming paradigm.
阅读全文
相关推荐
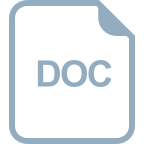
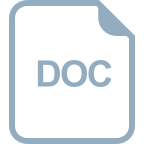
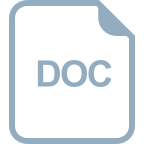
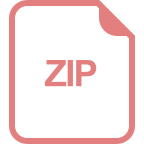
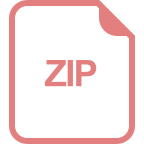
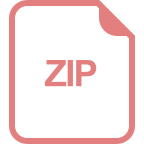
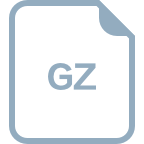
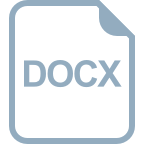
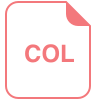
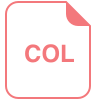
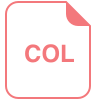
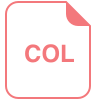
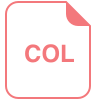




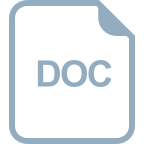