C++用for循环语句来分别求两个正整数的最大公约数和最小公倍数
时间: 2024-12-11 19:24:12 浏览: 10
在C++中,我们可以使用欧几里得算法(也称辗转相除法)来计算两个正整数的最大公约数(Greatest Common Divisor,GCD),然后利用最大公约数来找到最小公倍数(Least Common Multiple,LCM)。下面是使用for循环实现这两个操作的示例:
首先,计算GCD:
```cpp
int gcd(int a, int b) {
if (b == 0)
return a;
else
return gcd(b, a % b);
}
// 使用for循环优化递归过程
int gcdOptimized(int a, int b) {
int temp;
for (temp = a % b; b != 0; a = b, b = temp) {
temp = b;
}
return a;
}
```
接着,计算LCM:
```cpp
int lcm(int a, int b) {
return (a * b) / gcd(a, b);
}
```
你可以结合这两个函数,例如:
```cpp
int main() {
int num1, num2;
std::cout << "Enter two positive integers: ";
std::cin >> num1 >> num2;
// 计算并输出最大公约数
int gcdValue = gcdOptimized(num1, num2);
std::cout << "The GCD of " << num1 << " and " << num2 << " is: " << gcdValue << std::endl;
// 计算并输出最小公倍数
int lcmValue = lcm(num1, num2);
std::cout << "The LCM of " << num1 << " and " << num2 << " is: " << lcmValue << std::endl;
return 0;
}
```
阅读全文
相关推荐
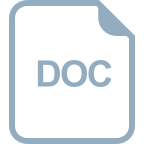
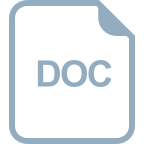
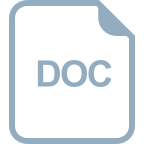





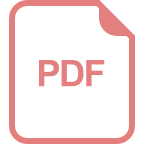
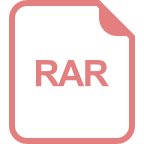
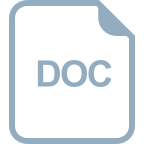
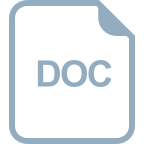


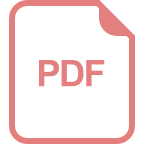
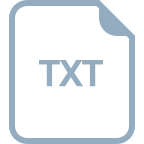
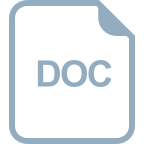
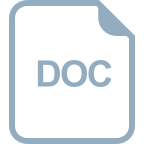
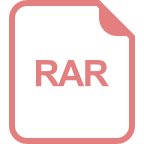